Vue Js Get character at a particular index in a string: The character at the given index is returned by the method charAt(). A string’s characters are indexed from left to right. In a string called stringName, the index of the first character is 0 and the index of the last character is string Name.In this example, we are going to explain how you can get the character of a string by using the charAt() feature in Vue.JS. You can try our “run online” feature and see the output live.
How to Use Vue Js charAt() method?
A new string containing the character at the passed-in index is returned by the String.charAt method. The charAt method returns an empty string if an index that you supply does not exist in the string. You can use charat() method in vue to get specified character of string simply as below
Vue Js Get character at a particular index in a string Example
<div id="app">
<button @click='myFunction'>click me </button>
<p>{{string}}</p>
<p>String Char: {{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
string: 'Fontawesomeicons',
results : ''
}
},
methods:{
myFunction(){
this.results = this.string.charAt(3);
},
}
}).mount('#app')
</script>
Output of above example
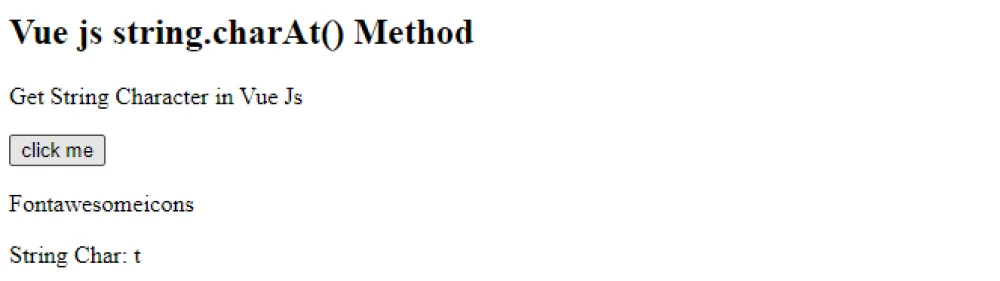
How to Get the First Character of a String in Vue Js?
In Vue.js, we’ll use the native javascript method charAt() on the string and pass it 0 as a parameter to retrieve the first character of the string: str.charAt (0). Using the character located at the specified index, the method returns a new string.
Vue js Get first character of String | Example
<div id="app">
<button @click='myFunction'>click me </button>
<p>{{string}}</p>
<p>String Char: {{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
string: 'Fontawesomeicons',
results : ''
}
},
methods:{
myFunction(){
this.results = this.string.charAt(0);
},
}
}).mount('#app')
</script>
Output of above example
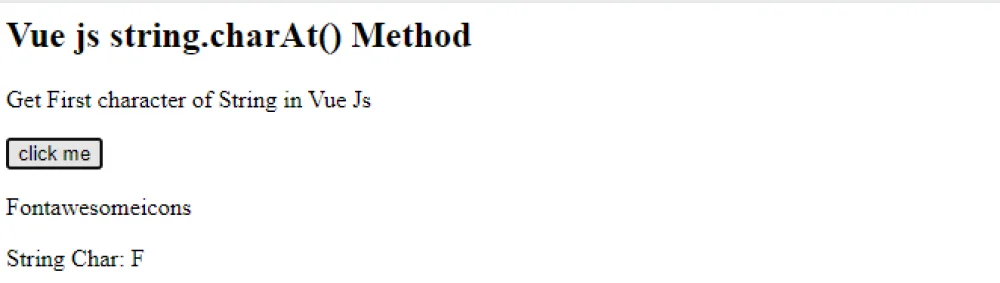
How to get the last character of a string in Vue Js?
Get the last string element in Vue.js Start by using the str.length function to determine the total number of characters in the given string. To obtain the last character of the string because indexing begins at 0, use str.charAt(str.length-1).
Vue Js get last element of String | Example
<div id="app">
<button @click='myFunction'>click me </button>
<p>{{string}}</p>
<p>String Char: {{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
string: 'Fontawesomeicons',
results : ''
}
},
methods:{
myFunction(){
this.results = this.string.charAt(this.string.length-1);
},
}
}).mount('#app')
</script>
Output of above example
