Vue Js String toUpperCase Method: To change a string into uppercase letters in Vue.js, you can use the JavaScript string toUpperCase() method. This method is built-in in JavaScript and can be used in Vue.js as well. It converts all the characters in a string to uppercase and returns the resulting string. To use it in Vue.js, simply call the toUpperCase() method on the string variable or property that you want to convert to uppercase, and store the result in a new variable or use it directly in your Vue.js template. This is a simple and straightforward way to change a string to uppercase in Vue.js.
How can I create an uppercase string in Vue.js?
The code is a simple example of using Vue.js, a JavaScript framework for building user interfaces, to convert a string to uppercase. Here’s a catchy explanation:
- The Vue.js app is defined inside a div with the ID “app”.
- There is a button with the text “click me”, which has a click event handler called “myFunction”.
- There are three paragraphs displaying text:
- “Convert string to Upper case in Vue Js”
- “Text:” followed by the value of the “text” data property
- “Upper Case:” followed by the value of the “results” data property
- Inside the Vue.js script:
- The “import” statement brings in the “createApp” function from the Vue.js library.
- The “createApp” function is called with an object that contains the app’s data and methods.
- The “data” method returns an object with two properties:
- “text” with the initial value of “font awesome icons”
- “results” with an empty string as the initial value
- The “methods” property contains a method called “myFunction” that converts the “text” data property to uppercase using the “toUpperCase” JavaScript string method and sets the result to the “results” data property.
- Finally, the “mount” method is called with the selector “#app” to attach the Vue.js app to the div with the ID “app” in the HTML code.
Example of Vue Js upper case method
<div id="app">
<button @click="myFunction">click me</button>
<p>Convert string to Upper case in Vue Js</p>
<p>Text:{{text}}</p>
<p>Upper Case :{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
text:'fontawesomeicons',
results:''
}
},
methods:{
myFunction(){
this.results = this.text.toUpperCase();
},
}
}).mount('#app')
</script>
Output of above example
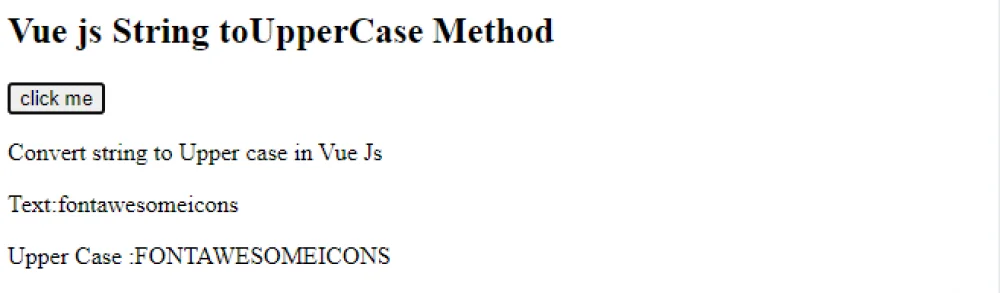
How is user input automatically uppercased by Vue.js?
The built-in JavaScript toUpperCase() function in Vue.js can be used to automatically uppercase user input.
Vue Js user input Upper case | Example
<div id="app">
<input type='text' placeholder='Enter Character' v-model="name" @input="myFunction"/>
<p>Upper Case :{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
name:'',
results:''
}
},
methods:{
myFunction(){
this.results = this.name.toUpperCase();
},
}
}).mount('#app')
</script>
Output of above example
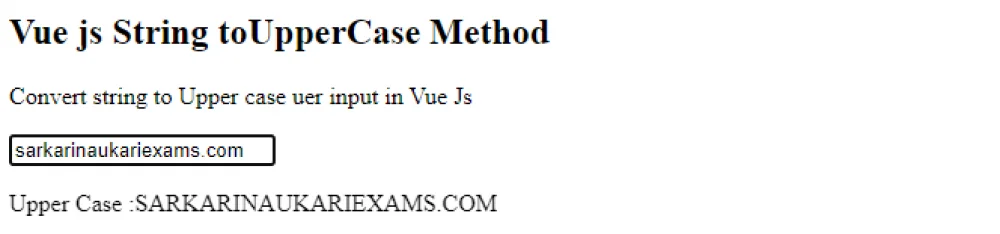
How to Capitalize a String’s First Letter in Vue.js?
To capitalise the first letter of a string in Vue: Get the first letter of the string using javascript’s built-in method “charAt,” which allows you to get the character at a specified index in the string, then capitalise the letter using the toUpperCase function, get the rest of the string using “slice” (1), and finally use the “concat” method to combine the first letter and the rest of the letter to get the result together.
Vue Js Capitalize first letter of string| Example
<div id="app">
<button @click="myFunction">click me</button>
<p>Convert string to Upper case in Vue Js</p>
<p>Text:{{text}}</p>
<p>First letter Captital: {{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
text:'fontawesomeicons',
firstLetter:'',
restLetter:'',
results:''
}
},
methods:{
myFunction(){
this.firstLetter = this.text.charAt().toUpperCase();
this.restLetter = this.text.slice(1);
this.results = this.firstLetter.concat(this.restLetter)
},
}
}).mount('#app')
</script>
Output of above example
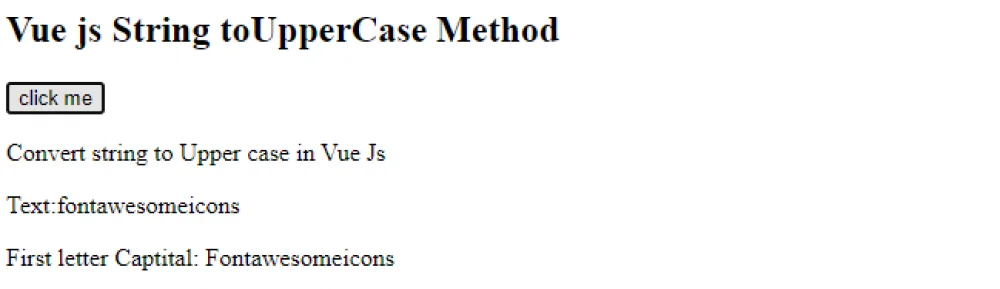