Vue Js Delete Table Row Dynamically: Deleting a row dynamically in Vue.js can be achieved by using the splice() method To delete a row dynamically in Vue.js, you can use the splice() method, which allows you to remove an item from an array With the click of a button, we can remove a table row from the display by referencing either its index or unique id. By clicking the button, a user can easily remove a table row from their HTML page without having to manually search for and delete the appropriate row. In this tutorial, we will learn how to delete a dynamic table row on a click button with the help of Vue JS and native JavaScript.
How to remove the table row in a table using Vue Js
Vue.js provides a v-on directive to listen to DOM events and run some JavaScript when they’re triggered Removing table rows on click can be achieved by using JavaScript to target the table row, and then utilizing the splice() method.
Vue Js Add or Remove table row Example
<div id="app">
<button @click="addRow" class="add-button">Add Row</button>
<br />
<table class="table">
<thead>
<tr>
<th>Row Number</th>
<th>Name</th>
<th>Number</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<tr v-for="(tableData, index) in tableRows" :key="index" :id="index" class="table-row">
<td>{{ index + 1 }}</td>
<td>
<input type="text" v-model="tableData.name" />
</td>
<td>
<input type="number" v-model="tableData.number" />
</td>
<td>
<button class="delete-button" @click="deleteRow(index)">Delete</button>
</td>
</tr>
</tbody>
</table>
</div>
<script type="module">
import { createApp } from "vue";
createApp({
data() {
return {
tableRows: [{ name: "Vue", number: 123 },
{ name: "React", number: 345 }
],
counter: 1,
};
},
methods: {
addRow() {
this.counter++;
this.tableRows.push({ name: "", number: "" });
},
deleteRow(index) {
this.tableRows.splice(index, 1);
},
},
}).mount("#app");
</script>
Output of Vue Js Add or Remove Table Row
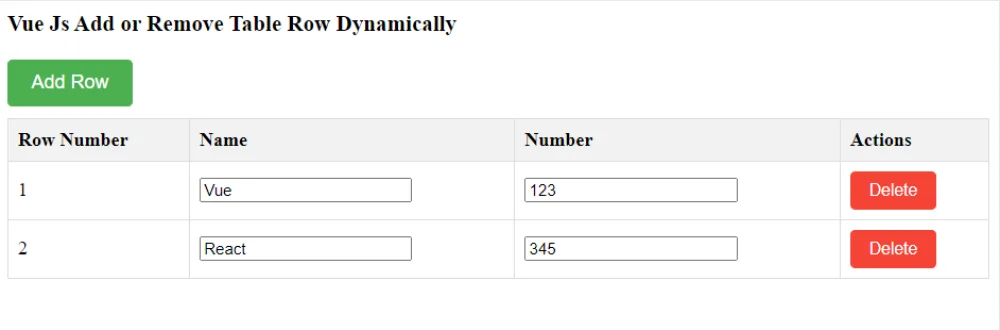
How can I remove or delete a table row in Vue ?
To remove or delete a table row in Vue.js, you can use the splice
method on the array that represents the table data. First, you need to identify the index of the row you want to delete, then call splice
on the array, passing in the index and a count of 1 to indicate that you want to remove one element starting at the index.
For example, if you have a data array called tableData
and you want to remove the row at index 2, you can call this.tableData.splice(2, 1)
in the method that handles the delete action. This will remove the row from the array and trigger Vue.js to re-render the table with the updated data.
Vue Remove Table Row Example
<div id="app">
<div class="table-container">
<h3>Vue Remove Add Row </h3>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Action</th>
</tr>
</thead>
<tbody>
<tr v-for="item in tableData" :key="item.id">
<td>{{ item.id }}</td>
<td>{{ item.name }}</td>
<td>
<button class="cancel-button" @click="removeRow(item.id)">Remove</button>
</td>
</tr>
</tbody>
</table>
</div>
</div>
<script type="module">
const app = Vue.createApp({
// Define the data properties and methods for the app
data() {
return {
tableData: [
{ id: 1, name: 'John' },
{ id: 2, name: 'Jane' },
{ id: 3, name: 'Bob' },
{ id: 4, name: 'Andrew' },
{ id: 5, name: 'James' },
{ id: 6, name: 'Sherlock' },
],
};
},
methods: {
removeRow(id) {
const index = this.tableData.findIndex(item => item.id === id);
if (index !== -1) {
this.tableData.splice(index, 1);
}
},
},
});
app.mount("#app");
</script>
Output of Vue Remove Table Row
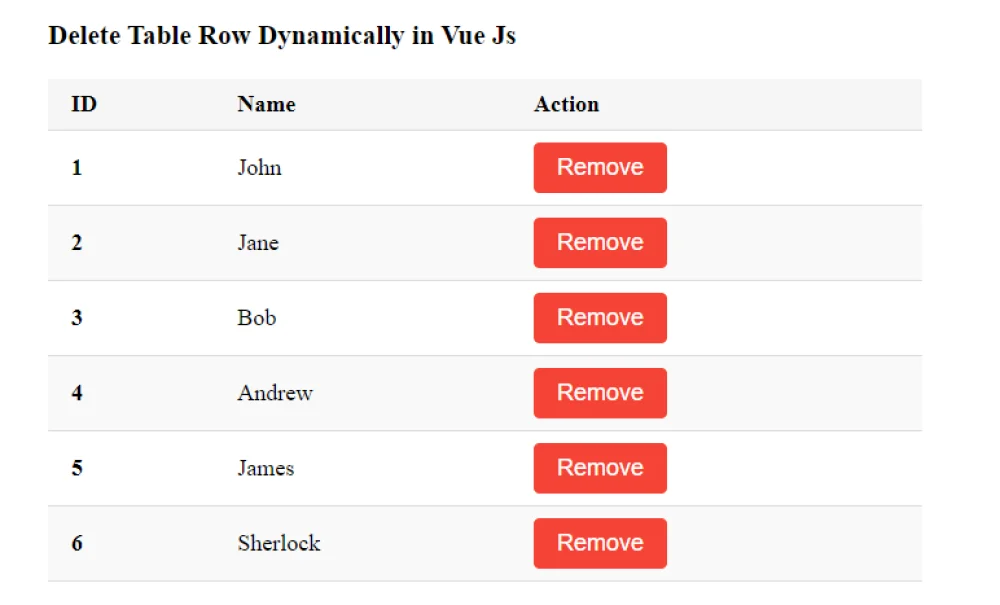