Vue Js Dropdown with Search Box : Dopdown with search functionality can be achieved by using computed properties to filter the options based on the user’s input in the search bar. Computed properties are a way to declaratively describe a value that depends on other values. They are cached based on their dependencies, so they are only updated when a dependency changes. The v-model directive is used to bind the value of the input field to a data property. This allows the value of the input field to be updated when the user types in the search bar, and it also allows the filtered options list to be updated when the search bar value changes. The v-on directive is used to listen for input events on the search bar and call a method when the input event is triggered. This method can be used to update the filtered options list based on the user’s input. The v-for directive is used to render the filtered options in the select element. It loops through the filtered options list and creates an option element for each item in the list.
By using these key concepts, you can create a dropdown with search functionality in Vue.js.
To create a dropdown with search functionality in Vue.js
we can use a combination of HTML, CSS, and JavaScript.
- Create a select element in your HTML template, with options for each item in your dropdown list.
- Add a search input field and bind it to a data property in your Vue instance using v-model.
- Use the computed property in Vue.js to filter the options based on the search input.
- Use the v-on directive to listen for input events on the search field, and update the filtered options list accordingly.
- Use v-for directive to render the filtered options in the select element.
Here is a sample Vue.js code that demonstrates the above steps:
Vue Js filter dropdown | Example
<div id="app">
<input style="width:250px" type="text" v-model="search" v-on:input="filterOptions" placeholder="Search"><br>
<select v-model="selectedOption" style="width:250px" v-bind:size="options.length">
<option disabled value="">Please select one</option>
<option v-for="option in filteredOptions" :value="option">{{ option }}</option>
</select>
<pre>Selected language: {{ selectedOption }}</pre>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
options: ['React', 'Vue', 'Angular', 'Node', 'Express', 'Bootstrap', 'MongoDb', 'Javascript', 'Vuetify'],
search: '',
selectedOption: '',
}
},
computed: {
filteredOptions() {
return this.options.filter(option => {
return option.toLowerCase().includes(this.search.toLowerCase());
});
}
},
methods: {
filterOptions() {
this.filteredOptions = this.options.filter(option => {
return option.toLowerCase().includes(this.search.toLowerCase());
});
},
}
});
</script>
Output of above example
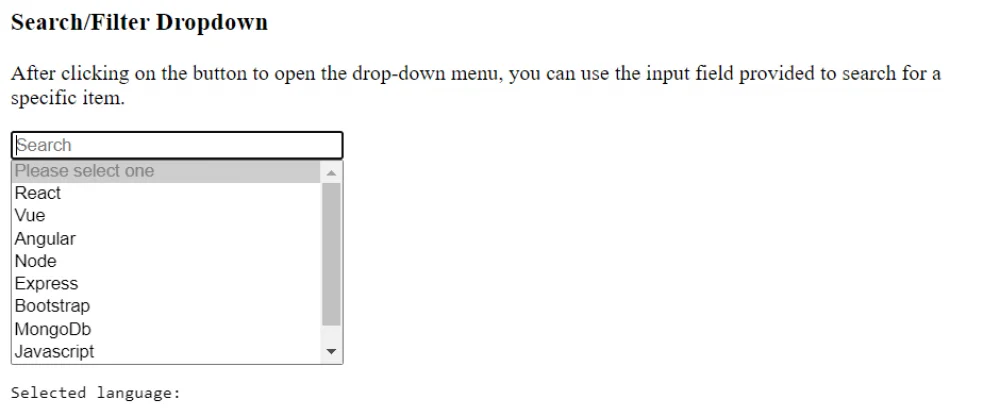