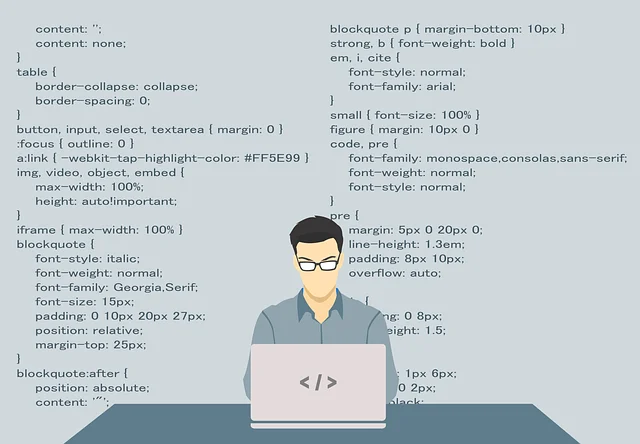
Vue Radio Button Default Checked/Selected: In Vue.js, the default value for a radio button can be set by binding the v-model
directive to a variable in the Vue instance’s data object, and setting the value of that variable to the value of the radio button that should be checked by default.
The v-model
directive creates a two-way data binding between the value of the radio button and the variable in the Vue instance’s data. When the radio button is selected, the value of the variable will be updated to match the value of the radio button, and when the value of the variable changes, the corresponding radio button will be selected.
By setting the value of the variable to the value of the radio button that should be checked by default, the corresponding radio button will be selected when the component is rendered.
How to set default selected radio button in Vue Js
In Vue.js, you can set a radio button as the default checked/selected option by binding the “checked” attribute to a data property and setting the initial value of that property to “true”.
This is a code snippet for a Vue.js application that displays a list of radio buttons with options for different programming languages. When a user selects one of the options, the selected value is stored in the Vue.js application’s data object and displayed in a “Selected Value” section below the radio buttons. The code uses Vue.js’ two-way data binding functionality to update the selected value in real-time. The app is initialized with the default selected value of “CSS”.
Vue Js Radio button default Checked/Selected Example
<div id="app">
<div class="radio-group" v-for='(option,index) in options'>
<input type="radio" :id="index" v-model="selectedValue" :value="option">
<label :for='index'>{{option}}</label>
</div>
<pre>Selected Value: {{selectedValue}}</pre>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
options: ['HTML', 'CSS', 'Javascript', 'Vue', 'Angular', 'React', 'Node'],
selectedValue: 'CSS'
}
},
});
</script>
Output of Vue Radio Button Default Checked
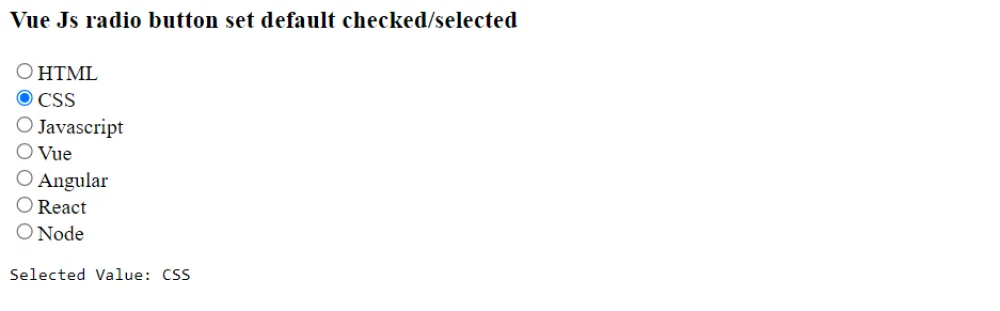