Vue Js Checked All/UnChecked All: In Vue.js, the v-model
directive can be used to bind the value of an input element to a data property in the component. When the input value changes, the bound data property is updated, and vice versa.
When it comes to a “select all” checkbox, you can have a separate checkbox that serves as the “select all” toggle. This checkbox can be bound to a data property in the component using the v-model
. When the value of this checkbox changes, you can use the watch
property in Vue to watch for changes to the data property and update the status of other checkboxes accordingly. In this tutorial, we will learn how to implement a “select all” and “unselect all” checkbox in Vue.js.
How to select all checkboxes by selecting one checkbox in Vue Js
In this example, the “checkAll” checkbox is bound to the checkedAll
property in the component’s data. When the user checks or unchecks the “check all” checkbox, the checkedAll
property is updated, and the other checkboxes are automatically selected or unselected through the use of v-model
. The watch
property is used to watch for changes to the selectAll
property and update the selected
property of each item accordingly.
Vue Js checkbox select all Example
<div id="app">
<input type="checkbox" v-model="checkedAll" />Checked All<br /><br />
<div v-for="(item,index) in items">
<input :value="item.language" type="checkbox" v-model="item.checked"/>{{item.language}}
</div>
</div>
<script type="module">
import { createApp } from "vue";
createApp({
data() {
return {
checkedAll: true,
items: [
{ language: "Vue", checked: true },
{ language: "React", checked: true },
{ language: "Angular", checked: true },
{ language: "Node", checked: true },
{ language: "Php", checked: true },
{ language: "Bootstrap", checked: true },
{ language: "Express", checked: true },
],
};
},
watch: {
checkedAll(value) {
this.items.forEach((item) => (item.checked= value));
},
},
}).mount("#app");
</script>
Output of above example
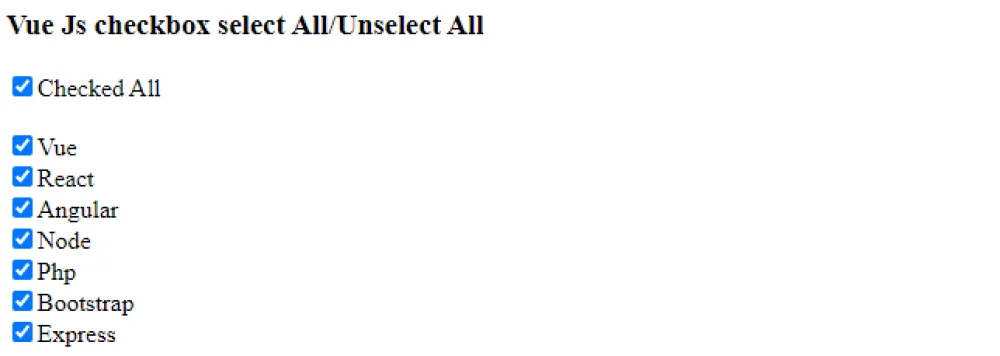