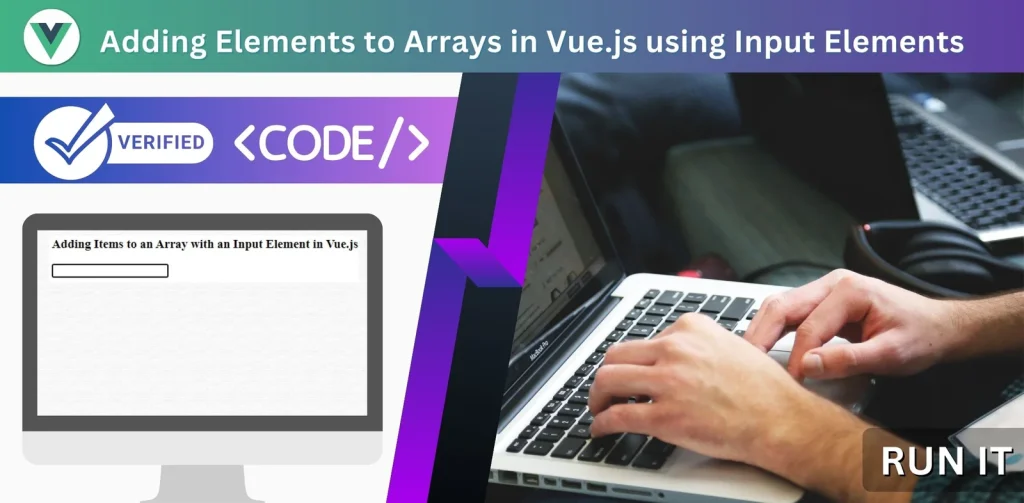
Vue Js Push items to an array with in Input element: In Vue, you can manipulate arrays to store and display data in your application. One common task is to push items to an array, which can be done in a number of ways.One of the most straightforward methods is to use an input element as the source of the item to be added to the array. This involves binding the input field to a data property using the v-model
directive. This allows the user to enter a value into the input field, which is then stored in the bound data property.
What is the process for adding items to an array in Vue.js using an input element?
The process for adding items to an array in Vue.js using an input element involves binding the input field to a data property using the v-model
directive, triggering a method with an event listener (such as @click
or @keyup.enter
) to add the item to the array, and utilizing the push
method of the array to add the item to the end of the list. The input field is used to capture the value entered by the user, which is then stored in the bound data property. When the event listener is triggered, the method runs and uses the push
method to add the value stored in the data property to the array.
Dynamic Array Management in Vue.js: Adding Elements with Input Elements
<div id="app">
<input v-model="newItem" @keyup.enter="addItem" />
<ul>
<li v-for="item in items" :key="item">{{ item }}</li>
</ul>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
newItem: '',
items: []
}
},
methods: {
addItem() {
this.items.push(this.newItem)
this.newItem = ''
}
}
});
</script>
Output of above example
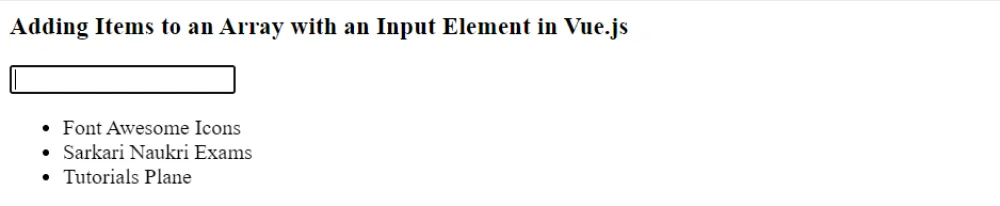