Dynamic Tab Creation and Management using Vue.js: VueJS Dynamic Tabs with Add and Remove Functionality refers to a feature in VueJS, a JavaScript framework for building user interfaces, where the tabs are generated dynamically and the user can add and remove tabs as needed. This feature is implemented using the data binding and reactive nature of VueJS to update the view whenever the underlying data changes. The tabs are typically stored in an array and each tab can be represented as an object with properties such as title and content. The add and remove functionality can be implemented using methods to push and splice the tabs array
How to implement Dynamic Tab Added or Remove functionality in Vue Js?
- Defines the data properties: The Vue instance has a data object with the following properties:
tabs
: an array of objects, each representing a tab with atitle
and abody
.activeTabIndex
: an integer representing the index of the currently active tab.
- Implements methods: The Vue instance has several methods for modifying the tabs:
setActiveTab
: sets the active tab index to the given index.addTab
: adds a new tab to thetabs
array with a defaulttitle
andbody
.removeTab
: removes the tab at the given index from thetabs
array.tabTitle
: returns the title of the tab at the given index.
- Renders the tabs: The Vue instance uses templates and Vue‘s reactive data and methods to dynamically render the tabs. The tabs are displayed in an unordered list (
ul
), and the active tab’s body is displayed in adiv
element. Theslot
directive is used to render the tab’s body. - Adds and removes tabs: The code implements buttons for adding and removing tabs. The
addTab
method is called when the user clicks the “+” button, and theremoveTab
method is called when the user clicks the “x” button (only visible when a tab is active).
Vue Js Dynamic Tab | Example
<div id="app">
<div class="tabs">
<ul class="tab-header">
<li v-for="(tab, index) in tabs" :key="index" :class="{ 'active-tab': activeTabIndex === index }"
@click="setActiveTab(index)">
{{ tabTitle(index) }}
</li>
<span class="add-remove-icons">
<span class="add-tab-icon" @click="addTab">+</span>
<span v-if="activeTabIndex !== null" class="remove-tab-icon"
@click="removeTab(activeTabIndex)">×</span>
</span>
</ul>
<div class="tab-body">
<slot v-for="(tab, index) in tabs">
<div v-if="activeTabIndex === index">
{{ tab.body }}
</div>
</slot>
</div>
</div>
</template>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
tabs: [
{
title: "Tab 1",
body: "This is the body of Tab 1"
}
],
activeTabIndex: 0
};
},
methods: {
setActiveTab(index) {
this.activeTabIndex = index;
},
addTab() {
const newTab = {
title: `Tab ${this.tabs.length + 1}`,
body: `This is the body of Tab ${this.tabs.length + 1}`
};
this.tabs.push(newTab);
this.activeTabIndex = this.tabs.length - 1;
},
removeTab(index) {
this.tabs.splice(index, 1);
this.activeTabIndex = this.tabs.length ? 0 : null;
},
tabTitle(index) {
return `Tab ${index + 1}`;
}
},
});
</script>
Output of above example
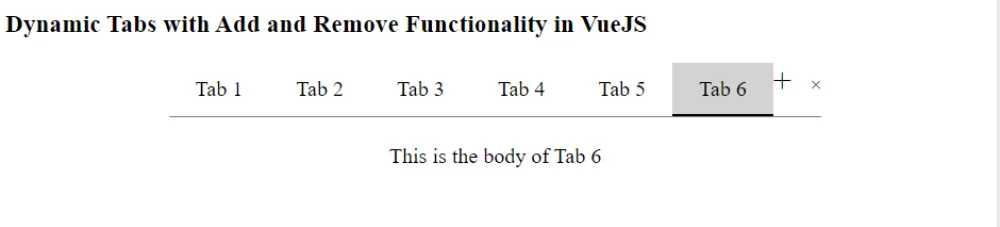