Dynamically Changing Textbox Color Based on Value in Vue JS: In Vue JS, you can dynamically change the color of a textbox based on its value using conditional classes. You can create a computed property that returns a class name based on the value of the textbox. For example, if the value is greater than 10, you can return a class name of ‘green’, otherwise, you can return a class name of ‘red’. Then, you can use the v-bind directive to bind the class attribute to the computed property. This will add the class to the textbox element dynamically based on its value, which will change its color according to the defined CSS styles for that class. By doing this, you can visually indicate to the user whether the textbox value is acceptable or not.
How can the background color of a textarea be changed dynamically based on its value using Vue.js?
This code uses Vue.js to dynamically change the background color of a textarea based on the length of its content. If the length of the content is greater than or equal to a predefined threshold value, the background color of the textarea is set to red. Otherwise, if the length is less than the threshold, the background color is set to green. The code achieves this by using a computed property called “inputClass” which returns an object with two properties, “red” and “green”, that are conditionally set based on the value of “value” and “threshold”. The CSS styles for the “red” and “green” classes are defined in the style section of the code.
Using Vue.js to dynamically change textbox color based on its value
<div id="app">
<textarea type="text" v-model="value" :class="inputClass"></textarea>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
value: '',
threshold: 10,
}
},
computed: {
inputClass() {
return {
'red': this.value.length >= this.threshold,
'green': this.value.length < this.threshold,
}
}
}
});
app.mount("#app");
</script>
<style>
.red {
background-color: #FF0000; /* Red */
}
.green {
background-color: #00FF00; /* Green */
}
</style>
Output of above example
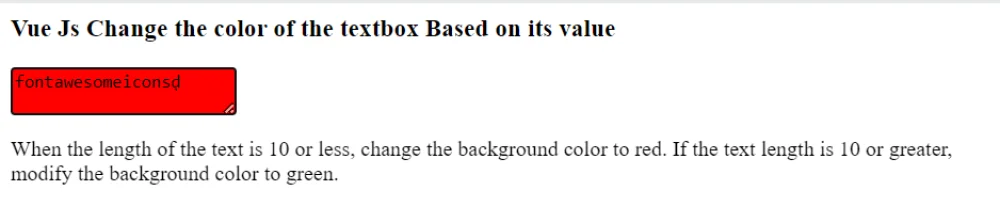