Vue Js Generate Random Color : Vue.js provides a shorthand syntax for binding style properties to data, which makes it easy to generate a random RGB color without any external libraries.In Vue.js, generating a random color can be accomplished using a combination of JavaScript’s built-in Math.random() function and a little bit of manipulation. The Math.random() function generates a random number between 0 and 1, which can be multiplied by 16777215 to get a random hexadecimal color code. This code can then be used to set the background color of an element or as a value for a CSS property. In order to ensure that the generated color is always a valid 6-digit hexadecimal code, the result should be padded with leading zeros if necessary. Overall, generating a random color in Vue.js is a simple task that can add a touch of interactivity and uniqueness to your applications.
How to implement Vue Js Random Hexa Decimal Color?
Here’s an explanation of the code to implement Vue Js Generate Random Hexa Decimal Color:
- The function
generateRandomColor()
is defined, which does not take any parameters. - Inside the function, a constant variable
randomColor
is declared using theconst
keyword. It is assigned the value of a randomly generated integer between 0 and 16777215 (inclusive), generated using theMath.random()
method and theMath.floor()
function. - The randomly generated integer is then converted to a string in base 16 (hexadecimal) using the
toString(16)
method. This returns a string representation of the number in hexadecimal format. - The string
randomColor
is then concatenated with the#
character to form a CSS color code, and the resulting color code is assigned to a property calledbgColor
. It is assumed thatthis
refers to the current object or component in which the function is being executed.
In summary, the generateRandomColor()
function generates a random hexadecimal color code and assigns it to the bgColor
property of the current object or component.
Vue Js Generate Random Color | Hexa Decimal Color Example
<div id="app">
<div :style="{ backgroundColor: bgColor, height: '100px', width: '100px' }"></div>
<p>{{bgColor}}</p>
<button @click="generateRandomColor">Generate Random Color</button>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
bgColor: '#f1f1f1'
}
},
methods: {
generateRandomColor() {
const randomColor = Math.floor(Math.random() * 16777215).toString(16);
this.bgColor = "#" + randomColor;
}
}
});
app.mount('#app');
</script>
Output of Vue Js Generate Random Color

How can I generate a random RGB color using Vue.js?
This JavaScript code defines a function called generateRandomColor()
that generates a random RGB color and sets it as the background color of an element. The function does the following:
- It first declares three variables,
r
,g
, andb
, and assigns them random values.Math.random()
generates a random decimal between 0 and 1.Math.floor()
rounds a decimal down to the nearest integer.- By multiplying the random decimal by 256, we get a random integer between 0 and 255, which is the range of valid RGB color values.
- The function then uses a template literal string to create an RGB color string with the values of
r
,g
, andb
interpolated into the string.- The template literal syntax
${}
is used to interpolate the variables into the string.
- The template literal syntax
- Finally, the function sets the
bgColor
property of the element to the generated color. This will change the background color of the element to the newly generated random color.
Overall, this function generates a random RGB color and sets it as the background color of an element. It is useful for adding some visual interest to a website or application by creating dynamic and ever-changing colors.
Vue Js Generate Random Color | RGB Color Example
<div id="app">
<div :style="{ backgroundColor: bgColor, height: '100px', width: '100px' }"></div>
<p>{{bgColor}}</p>
<button @click="generateRandomColor">Generate RGB Color</button>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
bgColor: 'rgb(225, 230, 93)'
}
},
methods: {
generateRandomColor() {
const r = Math.floor(Math.random() * 256);
const g = Math.floor(Math.random() * 256);
const b = Math.floor(Math.random() * 256);
this.bgColor = `rgb(${r}, ${g}, ${b})`;
}
}
});
app.mount('#app');
</script>
Output of Generate Random RGB Color
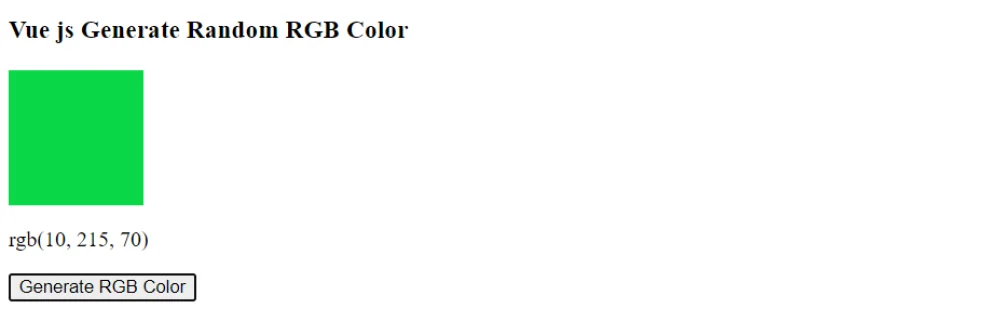