To update the width and height of an image with input field values in Vue.js, you can create two data properties in your Vue instance that will store the initial width and height values of the image. Then, you can bind these data properties to the width
and height
attributes of the img
tag using the v-bind
directive (:
shorthand).In this tutorial, we will learn how to update the width and height of an image using numerical input values.
What is the process to implement Vue.js to update the width and height of an image using the input field value?
This is a simple Vue.js application that allows you to adjust the width and height of an image using two input fields. The app starts by displaying an image with a default width and height of 500px and 300px respectively.
The input fields are created using HTML’s input
tag and v-model
directive binds the value of the input fields to the corresponding data properties in the Vue instance.
The :src
, :width
and :height
directives are used to bind the source, width, and height attributes of the img
tag to the corresponding data properties in the Vue instance. This means that when the user enters a new value for the image width or height, the corresponding property in the Vue instance is updated and the image is automatically resized to reflect the new dimensions.
Overall, this app demonstrates how Vue.js makes it easy to create reactive user interfaces that respond in real-time to user input.
Vue Js Update width and height of image using input field value example
<div id="app">
<div>
<label>Width:</label>
<input type="number" v-model="imageWidth">
</div>
<div>
<label>Height:</label>
<input type="number" v-model="imageHeight">
</div>
<img :src="url" :width="imageWidth" :height="imageHeight">
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
url: 'https://www.sarkarinaukriexams.com/images/post/1670771584desola-lanre-ologun-IgUR1iX0mqM-unsplash_(1).jpg',
imageWidth: 500,
imageHeight: 300,
}
},
});
app.mount('#app');
</script>
Output of above example
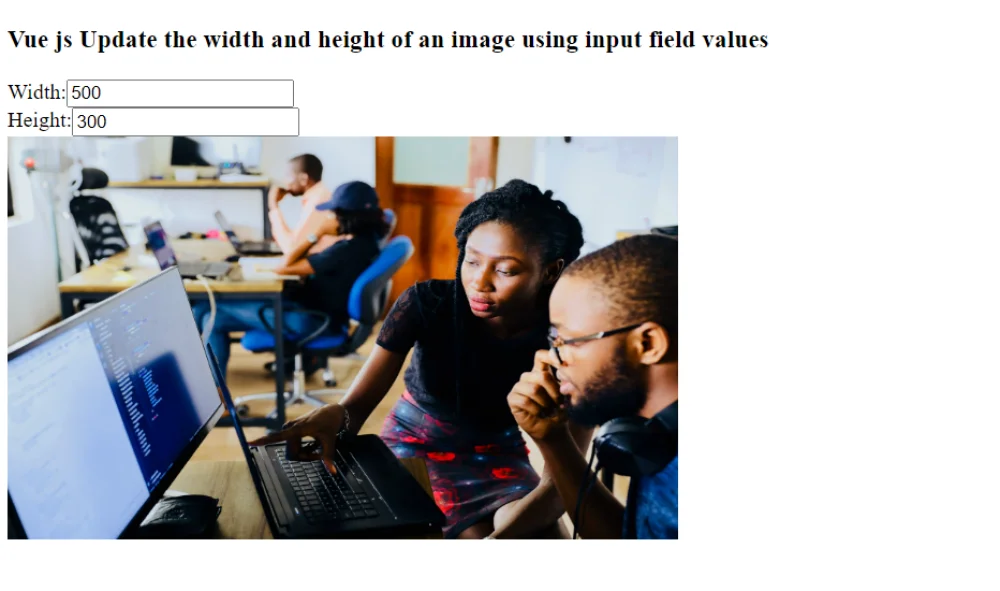