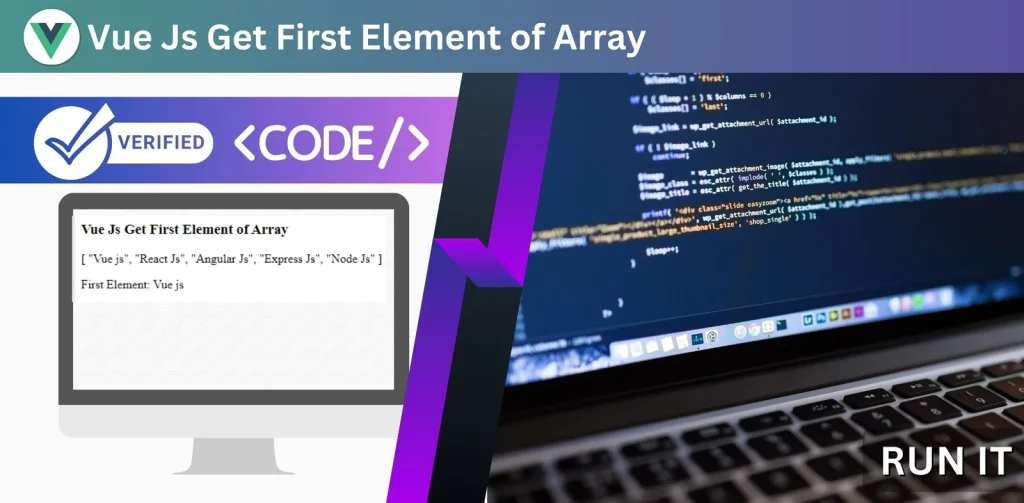
In this tutorial, we provide five examples to retrieve the first element of an array using
Vue.js. We employ indexing methods, the find method, slicing, and shifting. These methods are used to Get First Element of Array.
How to take first element of array in Vue Js?
This is the first example to obtain the first index of an array using Vue.js. In this example, we utilized the indexing method.
Vue Js Get First Element of Array using array indexing Example
<div id="app">
<p>{{arr}}</p>
<p>First Element: {{arr[0]}}</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
arr: ['Vue js', 'React Js', 'Angular Js', 'Express Js', 'Node Js'],
};
},
})
</script>
Output of above example

Example 2 : Vue Get First Element of List
In this example, we used a v-for loop with an index to retrieve the first value of an array with Vue.js
Vue v-for Only First Example
<div id="app">
<div v-for="(item, index) in items" :key="index">
<p v-if="index === 0">First element: {{ item }}</p>
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data: {
items: ['Apple', 'Banana', 'Orange', 'Grapes']
}
})
</script>
Output of above example

Example 3 : Vue Array Find Get First
This is the third method to get the first element, In this example we used the find method with Vue.js
Vue Js Get first element of array using find() Method Example
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
arr: ['Vue js', 'React Js', 'Angular Js', 'Express Js', 'Node Js'],
};
},
computed: {
firstElement() {
return this.arr.find((element, index, ) => index === 0);
}
}
})
</script>
Using the slice() Method to Retrieve the First Element of an Array in Vue.js
Vue Js Get first element using slice() method Example
<div id="app">
<p>{{arr}}</p>
<p>First Element: {{firstElement}}</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
arr: ['Vue js', 'React Js', 'Angular Js', 'Express Js', 'Node Js'],
};
},
computed: {
firstElement() {
return this.arr.slice(0, 1)[0];
}
}
})
</script>
Output of above example

Vue Js Get First Element of Array using array’s shift() method Example
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
arr: ['Vue js', 'React Js', 'Angular Js', 'Express Js', 'Node Js'],
firstElement: ''
};
},
mounted() {
this.firstElement = this.arr.shift()
}
})
</script>