Vue Js Find Number from String: In Vue.js, you can find a number from a string using regular expressions. Regular expressions are patterns used to match character combinations in strings. You can create a regular expression to match numbers within a string using the pattern “/\d+/” which will match one or more digits in the string. Once you have the matched digits, you can convert them to a number using the parseInt() function. For example, if you have a string “I have 10 apples”, you can use the regular expression pattern to match the number “10” and convert it to a number using parseInt().
How can extract a number from a string in Vue Js?
This code is written in JavaScript using the Vue.js framework, which is a popular library for building user interfaces. Here is a step-by-step explanation of Vue Js find Number from String:
- The code begins by defining a new Vue application using the
Vue.createApp()
method. This method creates a new instance of a Vue application, which we can then configure and mount to the DOM. - The
data()
method is used to define the initial state of our Vue application. In this case, we define two data properties:myString
andmyNumbers
.myString
is initialized with a string value that describes a quantity of fruit.myNumbers
is initialized as an empty array, which we will populate later with numeric values extracted frommyString
. - The
mounted()
method is a lifecycle hook that is called after the Vue instance has been mounted to the DOM. This is where we will put our code to extract numbers from themyString
property. In this case, we use a regular expression to find all numeric values in themyString
property and store them in themyNumbers
array. If no numeric values are found, we setmyNumbers
to an empty array.
Vue js Find Number from String Example
<div id="app">
<p>{{ myString }}</p>
<ul>
<li v-for="(number, index) in myNumbers" :key="index">{{ number }}</li>
</ul>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
myString: "I have 3 apples and 2 oranges.",
myNumbers: [],
}
},
mounted() {
this.myNumbers = this.myString.match(/\d+/g) || [];
},
});
app.mount('#app');
</script>
Output of Vue Js FInd Number from string
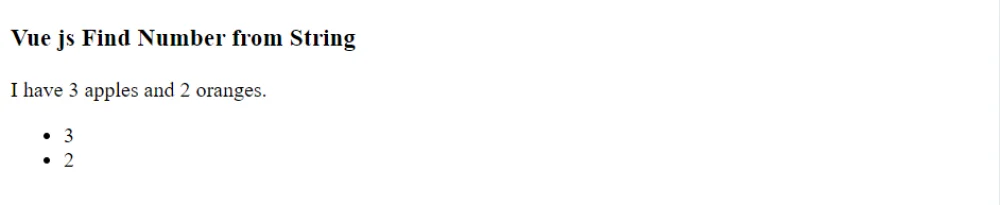