Vue Js Login Page:A Vue.js login form typically includes a username and password input fields, and a button to submit the form. The form should have validation rules to ensure that the inputs are valid before submitting the data. Once the form is submitted, the entered data is sent to the server for authentication. The server checks the credentials, and if they are valid, it responds with an authentication token. The token is then stored in the browser’s local storage, and the user is redirected to the authenticated portion of the app. If the credentials are invalid, the user is prompted to re-enter their credentials or may receive an error message. Overall, a Vue.js login form is a simple and essential component of any web application that requires user authentication.
Building a Secure Vue js Login Form for User Authentication
This is an HTML code snippet that creates a login form with email and password fields. The form is implemented using Vue js, a JavaScript framework for building user interfaces.
The login form consists of a div element with an id of “app” and a class of “login-container”. Inside this div, there is an h3 element with a class of “login-header” that displays the text “Log in”. Below the header, there is a form element with a class of “login-form” that is bound to a Vue method called “login” using the “@submit.prevent” directive. This method is executed when the form is submitted.
Inside the form, there are two form-group div elements, one for the email field and one for the password field. Each form-group div contains a label element and an input element. The input elements are bound to Vue data properties called “email” and “password” using the “v-model” directive. The input element for the password field also has a button element that toggles the visibility of the password by changing the input type between “password” and “text”.
The form also includes error handling for the email and password fields. If there are errors, a div with a class of “invalid-feedback” is displayed below the input field with the corresponding error message. Finally, there are two links below the form, one for signing up and one for resetting the password.
Vue Js Login Form | Page Example
<div id="app" class="login-container">
<h3 class="login-header">Log in</h3>
<form @submit.prevent="login" class="login-form">
<p class="signup-link">Need an account? <a href="#">Sign up</a></p>
<div class="form-group">
<label for="email" class="form-label">Email</label>
<input type="email" id="email" v-model="email" :class="{ 'is-invalid': errors.email }"
class="form-control form-input">
<div v-if="errors.email" class="invalid-feedback">{{ errors.email }}</div>
</div>
<div class="form-group">
<label for="password" class="form-label">Password</label>
<div class="password-input">
<input :type="showPassword ? 'text' : 'password'" id="password" v-model="password"
:class="{ 'is-invalid': errors.password }" class="form-control form-input">
<button type="button" class="password-toggle" @click="showPassword = !showPassword">{{ showPassword ? 'Hide' :
'Show' }}</button>
</div>
<div v-if="errors.password" class="invalid-feedback">{{ errors.password }}</div>
</div>
<button type="submit" class="btn btn-primary">Login</button>
<p class="forgot-password-link">Forgot your password? <a href="#">Click here</a></p>
</form>
</div>
Output of Vue Js Login Form | Page
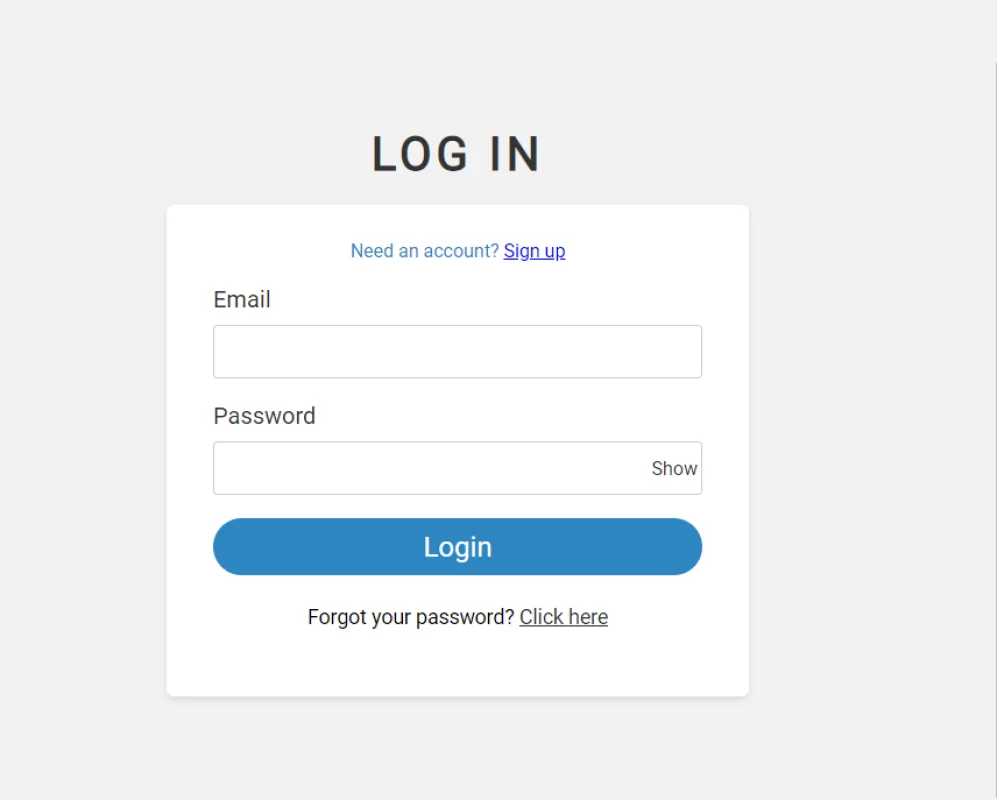
Creating a User Login Form with Vue js: Step-by-Step Script Code Tutorial
This is a Vue js application code that defines a Vue Js login form with email and password fields. The data
function returns an object with properties for the email and password inputs, an empty errors
object to track any validation errors, and a boolean showPassword
flag to toggle the password visibility.
The methods
property defines a login
function that sends a login request to the server with the provided email and password inputs. It first clears any previous errors in the errors
object, and then checks if the email and password fields are empty, adding any errors to the errors
object if necessary.
If there are no errors, the function checks if the provided email and password match a hardcoded set of credentials. If they do, it displays an alert with a success message. If not, it displays an alert with an error message.
Vue Js Login Form Script Code
<script type="module">
const app = Vue.createApp({
data() {
return {
email: '',
password: '',
errors: {},
showPassword: false
}
},
methods: {
login() {
// Reset errors
this.errors = {};
// Validate email
if (!this.email) {
this.errors.email = 'Email is required.';
} else if (!/\S+@\S+\.\S+/.test(this.email)) {
this.errors.email = 'Invalid email format.';
}
// Validate password
if (!this.password) {
this.errors.password = 'Password is required.';
} else if (this.password.length < 8) {
this.errors.password = 'Password must be at least 8 characters long.';
}
// If no errors, submit form
if (Object.keys(this.errors).length === 0) {
// Send login request to server with username and password
// If successful, redirect to home page
// If unsuccessful, display error message
if (this.email === 'xyz@gmail.com' && this.password === 'mypassword') {
// Replace with your own logic to handle successful login
alert('Logged in successfully!');
} else {
alert('Invalid email or password.');
}
}
}
},
});
app.mount("#app");
</script>
Styling Your Vue js Login Form: Essential CSS Code for a Polished User Interface
This is a CSS style sheet containing custom styles for a Vue Js login form. It defines custom fonts, colors, and styles for the form container, header, input fields, submit button, and error messages. The form is set up using flexbox to align and position the elements. The input fields have a hover effect and a password toggle feature. The submit button has a hover effect and a rounded border. The error messages are styled with a red color and the input fields are marked as invalid with a red border. The form also includes a link to a forgot password feature. Overall, the style sheet creates a modern and user-friendly Vue Js login form.
Vue Js Login Form CSS Code
<style>
/* Use a custom font */
@import url('https://fonts.googleapis.com/css2?family=Roboto&display=swap');
/* Define colors */
:root {
--primary-color: #2E86C1;
--danger-color: #dc3545;
--gray-color: #f2f2f2;
}
/* Set base styles */
* {
box-sizing: border-box;
font-family: 'Roboto', sans-serif;
}
body {
margin: 0;
}
/* Style the container */
.login-container {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
background-color: var(--gray-color);
}
/* Style the form */
.login-form {
display: flex;
flex-direction: column;
align-items: center;
width: 100%;
max-width: 400px;
padding: 2rem;
background-color: white;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
}
/* Style the form header */
.login-header {
font-size: 2rem;
margin-bottom: 1rem;
color: #333;
text-align: center;
text-transform: uppercase;
letter-spacing: 0.1em;
font-weight: bold;
}
/* Style the form input */
.form-group {
display: flex;
flex-direction: column;
width: 100%;
margin-bottom: 1rem;
}
.form-label {
font-size: 1rem;
margin-bottom: 0.5rem;
color: #333;
}
.signup-link {
font-size: 0.8rem;
margin-top: -0.5rem;
margin-bottom: 1rem;
text-align: right;
color: var(--primary-color);
text-decoration: none;
}
.signup-link:hover {
text-decoration: underline;
}
.form-control {
width: 100%;
padding: 0.5rem;
font-size: 1rem;
border-radius: 3px;
border: 1px solid #ccc;
transition: all 0.2s ease;
}
.form-control:focus {
border-color: var(--primary-color);
box-shadow: 0 0 0 0.2rem rgba(0, 123, 255, 0.25);
}
.password-input {
position: relative;
}
.password-toggle {
position: absolute;
top: 50%;
right: 0;
transform: translateY(-50%);
font-size: 0.8rem;
padding: 0.2rem;
background-color: transparent;
border: none;
cursor: pointer;
outline: none;
color: #333;
}
.password-toggle:hover {
text-decoration: underline;
}
/* Style the submit button */
.btn {
padding: 0.5rem 1rem;
/* increase padding to make the button bigger */
font-size: 1.2rem;
/* increase font size */
border-radius: 50px;
/* use a larger value for border-radius to make the button more rounded */
border: none;
color: white;
background-color: var(--primary-color);
cursor: pointer;
transition: all 0.2s ease;
width: 100%;
}
.btn:hover {
background-color: #0069d9;
}
/* Style the error message */
.is-invalid {
border-color: var(--danger-color) !important;
}
.invalid-feedback {
font-size: 0.8rem;
color: var(--danger-color);
}
.forgot-password-link {
margin-top: 20px;
text-align: center;
font-size: 14px;
}
.forgot-password-link a {
color: #333;
text-decoration: underline;
}
.forgot-password-link a:hover {
text-decoration: none;
}
</style>