Vue Table Sticky header:Vue Table Sticky Header or Table Header Fixed is a feature that allows the header of a table to remain visible while scrolling through its content. It is particularly useful when working with large datasets that require scrolling to access all the data. By fixing the header, users can easily refer to the column names and data types as they navigate through the table. This feature is implemented through CSS positioning and JavaScript calculations, which keep the header fixed to the top of the screen while allowing the content to scroll beneath it. Overall, it is a simple but effective way to enhance the usability of tables in Vue applications.
How can I implement a sticky header in a table using Vue.js?
To implement a sticky header in a table using Vue.js, you can first create a CSS class for the table container with a fixed height and “overflow: auto” property to enable scrolling. Then, add a “position: sticky” property to the <thead> element and set the “top” value to 0. This will make the header stick to the top of the table container as the user scrolls down. Finally, add a “z-index” property to ensure that the header stays on top of the table body. This can be achieved by adding the above CSS to your Vue.js component’s template or a separate stylesheet.
Vue Table Sticky header Example
<div id="app">
<div class="table-container">
<table>
<thead>
<tr>
<th v-for="header in headers" :key="header">{{ header }}</th>
</tr>
</thead>
<tbody>
<tr v-for="(row, index) in rows" :key="index">
<td v-for="cell in row" :key="cell">{{ cell }}</td>
</tr>
</tbody>
</table>
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
headers: ['Name', 'Age', 'Email'],
rows: [
['John', 30, 'john@example.com'],
['Jane', 25, 'jane@example.com'],
],
};
},
});
</script>
<style scoped>
.table-container {
max-width: 1200px;
margin: 0 auto;
position: relative;
height: 400px;
overflow: auto;
}
/* Style table */
table {
width: 100%;
border-collapse: collapse;
margin-bottom: 1em;
}
thead {
position: sticky;
top: 0;
background-color: #fff;
z-index: 1;
}
thead th {
background-color: #f5f5f5;
text-align: left;
padding: 0.5em 1em;
border-bottom: 1px solid #ddd;
}
tbody tr:nth-child(even) {
background-color: #f9f9f9;
}
td {
padding: 0.5em 1em;
border-bottom: 1px solid #ddd;
}
td:first-child {
font-weight: bold;
}
</style>
Output of Vue Table Sticky header
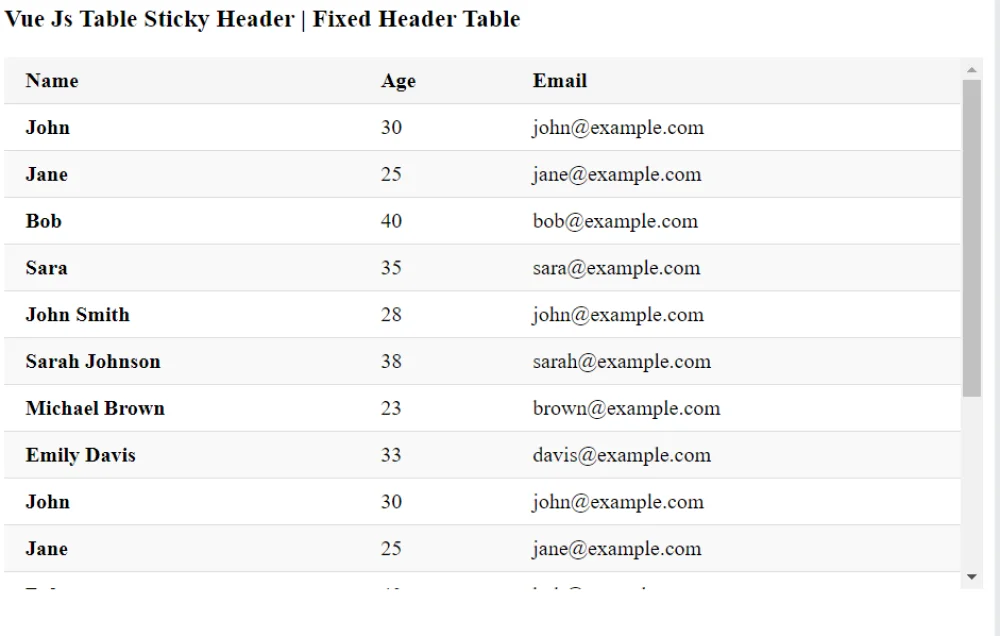