Vue Add Remove Input Field:Vue Add Remove Input Field is a common feature in many forms where users can add or remove input fields dynamically. This feature allows users to dynamically add or remove input fields based on their needs, which helps to reduce clutter and make the form more user-friendly. In Vue, this can be achieved by using a combination of v-for directive to render a list of input fields, and v-model directive to bind each input field’s value to a data property. Adding and removing input fields can be done by manipulating the data array. A common approach is to add a button to add new input fields, and an “X” button next to each input field to remove it.
How can you dynamically Vue add and remove input fields?
The code below is a Vue.js implementation of dynamically adding and removing input fields. It uses a v-for directive to loop through the fields array and render an input field and a remove button for each field. The addField() method is called when the user clicks the “Add Field” button, which adds an empty string to the fields array, and a new input field is rendered. The removeField() method is called when the user clicks the “Remove” button for a specific field, which removes the corresponding field from the fields array and removes the input field from the DOM. This allows for a dynamic and flexible form input system.
Vue Add Remove Input Field Example
<div id="app">
<div v-for="(field, index) in fields" :key="index" class="field-wrapper">
<input type="text" v-model="fields[index]" />
<button @click="removeField(index)">Remove</button>
</div>
<button @click="addField()" class="add-button">Add Field</button>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
fields: [''] // initial value
};
},
methods: {
addField() {
this.fields.push(''); // add empty value to the fields array
},
removeField(index) {
this.fields.splice(index, 1); // remove field at the specified index
}
}
});
</script>
Output of Vue Add Remove Input Field
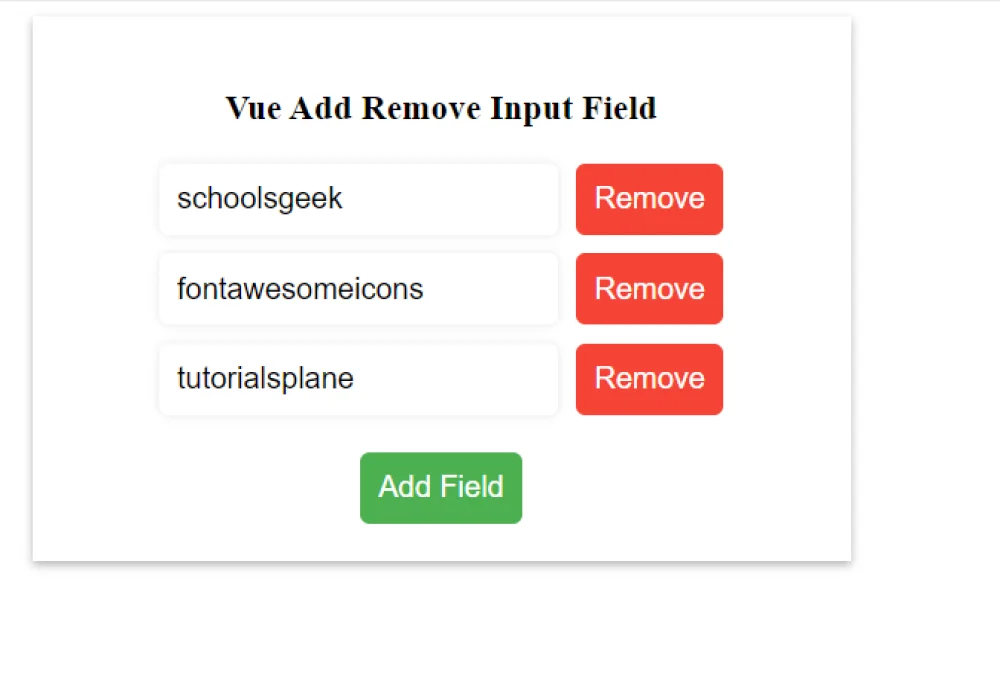