Vue Remove item from array by key index: In Vue, the splice method is used to modify an array by adding or removing elements. To remove an item from an array by its key index, you can use this method with two arguments. The first argument is the index at which to start changing the array, and the second argument is the number of elements to remove. To remove a single item at a specific index, you only need to pass that index as the first argument and 1 as the second argument. This will remove the item at the specified index and shift the remaining items to fill the gap. By using the splice method, you can easily manipulate arrays in Vue.js.
How can I remove an item from a Vue js array using the key index of that item?
This code is a Vue.js application that displays a list of items with their names and descriptions. Each item in the list has a delete button next to it that, when clicked, removes the item from the list using the splice
method. The splice
method takes two arguments – the index of the item to remove and the number of items to remove (in this case, just one). The deleteItem
method is called when the delete button is clicked and passes in the index of the item to be removed. The items
array is stored in the data
object of the Vue instance, and the list is generated using a v-for
directive that loops through the items and displays their names and descriptions. The key
attribute is used to uniquely identify each item in the list and improve performance when updating the list
Vue Remove item from array by key index Example
<div id="app">
<ul>
<li v-for="(item, index) in items" :key="index">
<span>{{ item.name }}</span>
<span>{{ item.description }}</span>
<button @click="deleteItem(index)">Delete</button>
</li>
</ul>
</div>
<script>
const app = Vue.createApp({
data() {
return {
items: [
{ name: 'Item 1', description: 'Description 1' },
{ name: 'Item 2', description: 'Description 2' },
{ name: 'Item 3', description: 'Description 3' }
],
};
},
methods: {
deleteItem(index) {
this.items.splice(index, 1);
},
},
});
app.mount('#app');
</script>
Output of Vue Js Remove item from Array by Index
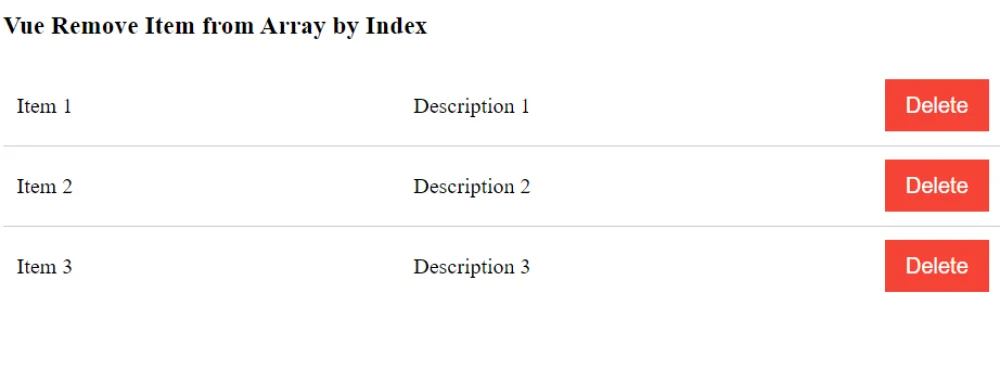