Vue Js Filter array of Object: To create a filter/search array in Vue.js, you can start by defining a data array in your Vue instance that contains the items you want to filter. Then, you can create a computed property that returns a filtered version of that array based on a search query input by the user.
The computed property can use the filter()
method to create a new array that includes only the items that match the search query. The search query can be stored in a data property and updated using a v-model directive on an input field.
To implement the filter functionality, you can use a v-for directive to loop through the computed array and display the filtered items in your template.
Overall, creating a filter/search array in Vue.js involves defining a data array, creating a computed property that filters the array based on user input, and using a v-for directive to display the filtered items in your template.
How to generate an array filter/search in Vue.js?
This code is an example of how to generate an array filter/search in Vue.js.
It starts with creating a data object that includes an array of items and a searchText variable that will hold the text the user is searching for.
Then, a computed property is used to generate a new array of filtered items based on the search text. The computed property uses the filter method on the items array to check if the item name or category includes the search text.
Finally, the filtered items are displayed in the HTML using a v-for loop. If there are no filtered items, a message is displayed indicating that no items were found.
Vue Js Filter array of Object Example
<div id="app">
<div class="search-box">
<label for="search">Search:</label>
<input type="text" id="search" v-model="searchText">
</div>
<ul class="item-list">
<li v-for="item in filteredItems" :key="item.name">
{{ item.name }} - {{ item.category }}
</li>
<li v-if="filteredItems.length === 0">
No items found.
</li>
</ul>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
items: [
{ name: "Apple", category: "Fruit" },
{ name: "Banana", category: "Fruit" },
{ name: "Carrot", category: "Vegetable" },
{ name: "Broccoli", category: "Vegetable" }
],
searchText: ""
};
},
computed: {
filteredItems() {
return this.items.filter(item => {
return (
item.name.toLowerCase().includes(this.searchText.toLowerCase()) ||
item.category.toLowerCase().includes(this.searchText.toLowerCase())
);
});
}
}
})
app.mount('#app')
</script>
Output of Vue Js Filter Array of Object
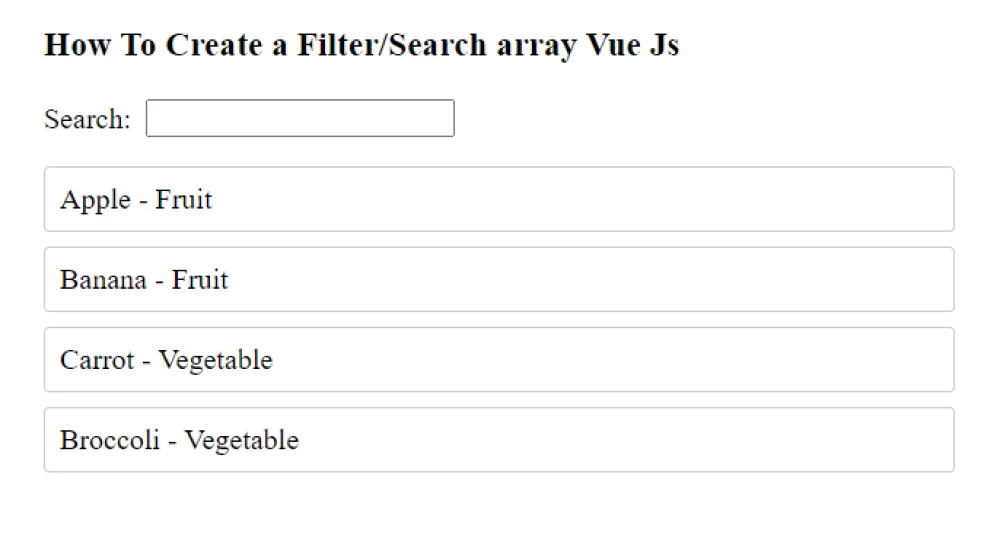