Vue Get Element By Key:In Vue.js, you can retrieve a specific element in a list by its unique identifier or key using the $refs
property. This is useful if you need to manipulate or access a specific element in the list, such as when you want to update its data or trigger an event on it. To use $refs
, you need to assign a ref to the element when you define it in your template. Then, you can access the element by its ref name and key using the $refs
property. Keep in mind that this should be used sparingly and as a last resort, as manipulating the DOM directly in Vue can lead to unexpected behavior.
How can you retrieve a specific element in a Vue.js component using its unique key?
This is a Vue.js component that renders a list of items using a v-for
directive. Each item is wrapped in a div
element with a unique key
attribute based on its index. The component also has a mounted
lifecycle hook that retrieves the second element using a ref
attribute, and updates a data property with its content. The updated data is then rendered in a paragraph element using Vue’s template syntax.
Vue Get Element By Key Exampe
<div id="app">
<div v-for="(item, index) in items" :key="index" ref="myElements">
{{ item }}
</div>
<p>Second Element: {{result}}</p>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
items: ['item1', 'item2', 'item3'],
result: ''
}
},
mounted() {
const secondElement = this.$refs.myElements[1]
this.result = secondElement.innerHTML
}
})
app.mount('#app')
</script>
Output of Vue Get Element By Key
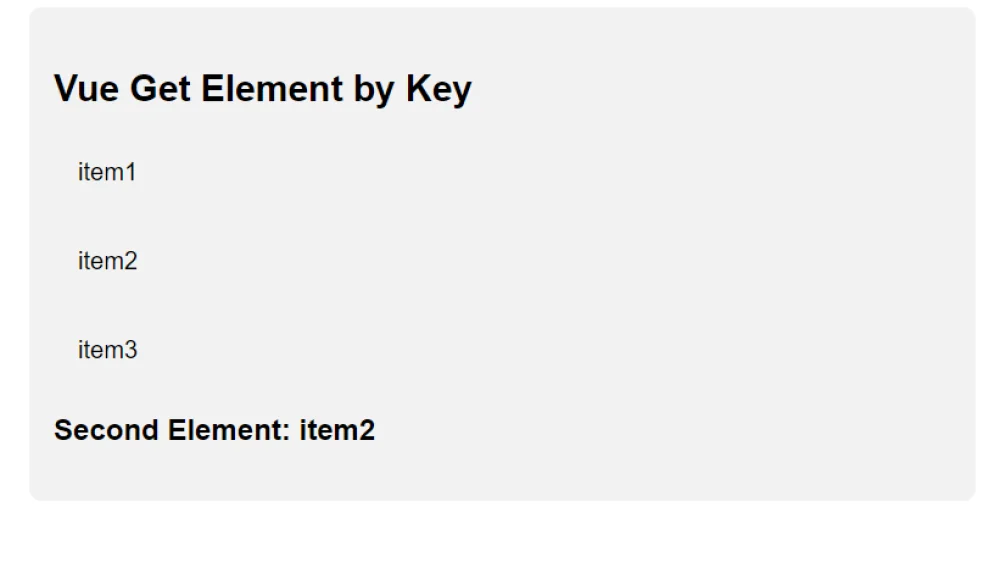