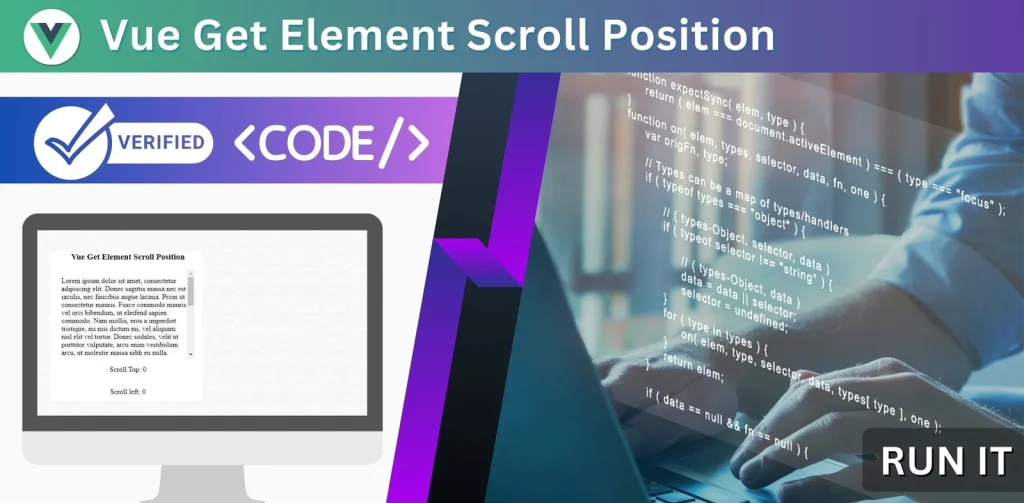
Vue Get Element Scroll Position:To get the scroll position of an element in Vue.js, you can use the scrollTop
property. This property is used to get or set the number of pixels an element is scrolled from its top position.
First, you need to get a reference to the element using the ref
attribute. Then, you can access the scrollTop
property of the element using this.$refs.element.scrollTop
in the Vue component’s methods or computed properties.
You can also add an event listener to the element to listen for scroll events using the @scroll
directive, which will trigger a method to update the scroll position. With these techniques, you can easily get and track the scroll position of an element in Vue.js
What is the method to get the scroll position of an element in Vue?
This code demonstrates how to get the scroll position of an element in Vue. The <div>
with id="app"
contains a nested <div>
element with a specified height and width, and the overflow
property set to auto
to enable scrolling.
The Vue app is created using Vue.createApp()
and includes a data
object with two properties, positionTop
and positionLeft
, both initialized to 0
. The mounted()
lifecycle hook is used to attach an event listener to the nested <div>
element with a reference of myElement
.
The event listener listens for the 'scroll'
event and updates the positionTop
and positionLeft
properties in the data
object with the current scroll position of the element using el.scrollTop
and el.scrollLeft
, respectively. These properties are then displayed in two separate <p>
tags below the <div>
element.
Overall, this code demonstrates how to use Vue’s reactivity system to update the view based on the user’s interaction with the page, specifically the scrolling of a specific element.
Vue Get Element Scroll Position Example
<div id="app">
<h3>Vue Get Element Scroll Position</h3>
<div ref="myElement" style="height:200px; width:300px; overflow:auto;">
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Donec sagittis massa nec est iaculis, nec
faucibus augue lacinia. Proin ut consectetur mauris. Fusce commodo mauris vel orci bibendum, ut eleifend
sapien commodo. Nam mollis, eros a imperdiet tristique, mi nisi dictum mi, vel aliquam nisl elit vel
tortor. Donec sodales, velit ut porttitor vulputate, arcu enim vestibulum arcu, ut molestie massa nibh
eu nulla.</p>
<p>Nulla id magna auctor, posuere velit quis, dictum tellus. Duis sed ullamcorper nunc, euismod euismod
sapien. Etiam eleifend vestibulum nisi, eu dictum erat dignissim eget. Nullam efficitur mi vel risus
ultricies vestibulum. Pellentesque consequat velit ac arcu dignissim, vel fermentum justo viverra.
Aenean laoreet pretium ante non pharetra. Praesent gravida eget purus id feugiat. Aliquam sit amet
turpis sapien. Suspendisse interdum volutpat odio vel semper. Sed sed tellus nec dolor bibendum
elementum. Etiam vel augue ex. Etiam venenatis, nisi non pulvinar pharetra, ex magna feugiat risus, ac
auctor justo nibh vel nisl.</p>
</div>
<p>Scroll Top: {{positionTop}}</p>
<p>Scroll left: {{positionLeft}}</p>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
positionTop: 0,
positiontLeft: 0
}
},
mounted() {
const el = this.$refs.myElement;
el.addEventListener('scroll', () => {
this.positionTop = el.scrollTop;
this.positionLeft = el.scrollLeft;
});
},
})
app.mount('#app')
</script>
Output of Vue Get Element Scroll Position
