Vue Element Resize Event:Vue Element Resize Event is a plugin that allows you to detect when an element has been resized. It provides a directive that can be applied to any element, and it emits a “resize” event whenever the element’s size changes. This can be useful for dynamically adjusting the layout of your application in response to changes in the size of the browser window or the size of a parent element. The plugin uses the ResizeObserver API to detect changes in size, which is supported in modern browsers.
How can I detect when an element’s size changes in Vue js?
This code is a simple Vue.js app that detects changes to the size of a container element and updates the displayed width.
It does this by using the ref
attribute to create a reference to the container element, which can then be accessed in the mounted
lifecycle hook using this.$refs.container
. The initial width is set in mounted
using this.containerWidth = this.$refs.container.offsetWidth
.
A handleResize
method is defined to update the containerWidth
whenever the window is resized. This method is attached as an event listener in mounted
using window.addEventListener('resize', this.handleResize)
, and removed in beforeDestroy
using window.removeEventListener('resize', this.handleResize)
to avoid memory leaks.
The width of the container is displayed in the template using interpolation syntax, like this: {{containerWidth}}
.
Vue Element Resize Event Example
<div id="app">
<div ref="container" class="container">
<p>Resize the window to see the effect</p>
<pre>Container width: {{containerWidth}}</pre>
</div>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
containerWidth: null
}
},
mounted() {
this.containerWidth = this.$refs.container.offsetWidth
window.addEventListener('resize', this.handleResize)
},
beforeDestroy() {
window.removeEventListener('resize', this.handleResize)
},
methods: {
handleResize() {
this.containerWidth = this.$refs.container.offsetWidth
}
}
})
app.mount('#app')
</script>
Output of Vue Element Resize Event
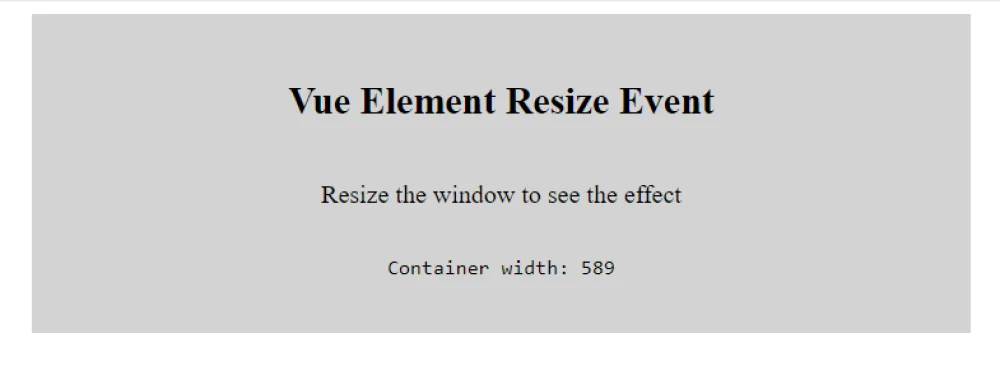