Vue Input get focus event invalid:In Vue, the ref attribute allows you to create a reference to a child component or element in a parent component. By combining the ref attribute with event listeners, you can programmatically give focus to an input element when it becomes invalid.
First, you would add a ref attribute to the input element that you want to target. Then, you can add an event listener to the input element to listen for when it becomes invalid. When the input becomes invalid, you can use the ref to access the input element and call its focus() method to give it focus. This allows the user to easily correct the invalid input without having to manually click on the input element.
How can you make a Vue input element automatically gain focus when an invalid input event occurs?
This is a Vue app that contains a form with a password input field and a submit button. The password input field is bound to the Vue app’s data using v-model
. There is also a label for the input field and an error message that is displayed when the input is invalid.
The validateInput
method is called when the user clicks the submit button. This method checks if the input is valid by checking if it is at least 6 characters long. If it is not valid, the method sets isInvalid
to true and focuses the password input field using $refs.myInput.focus()
. If the input is valid, isInvalid
is set to false.
Vue Input get focus event invalid Example
<div id="app">
<label for="my-input">Enter a password:</label>
<input id="my-input" ref="myInput" type="password" v-model="inputValue" :class="{ 'invalid': isInvalid }">
<div v-if="isInvalid" class="error-message">Password must be at least 6 characters long.</div>
<button @click="validateInput">Submit</button>
</div>
<script>
const app = Vue.createApp({
data() {
return {
inputValue: '',
isInvalid: false
}
},
methods: {
validateInput() {
const minLength = 6;
if (this.inputValue.length < minLength) {
this.$refs.myInput.focus();
this.isInvalid = true;
} else {
this.isInvalid = false;
}
}
}
});
app.mount('#app');
</script>
Output of Vue Input get focus event invalid
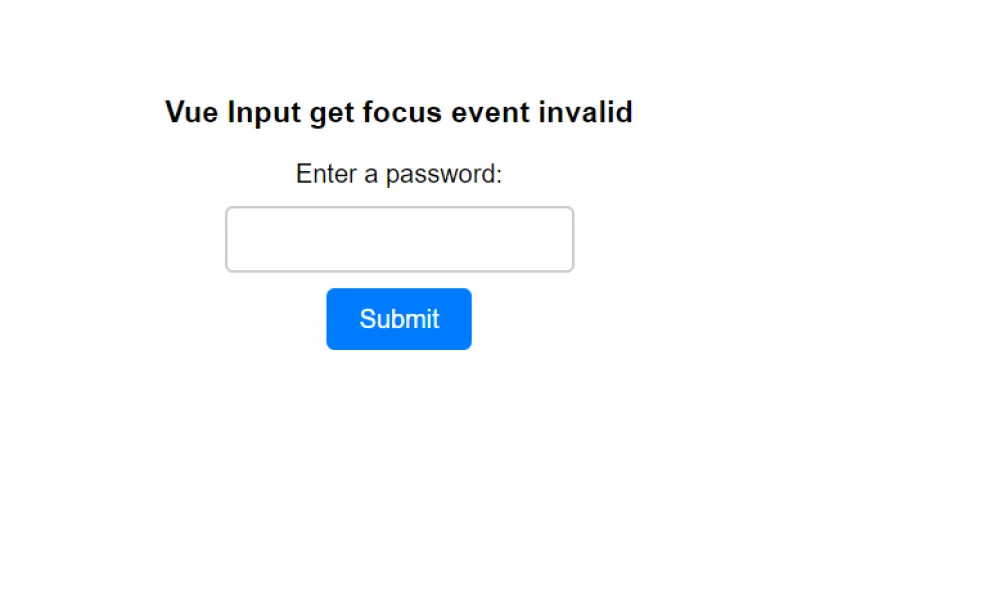