Vue Js Disable input Field Conditionally:In Vue, you can disable an input field conditionally by binding the disabled
attribute to a Boolean expression that evaluates to true or false using the ternary operator.
For instance, let’s say you have a data property called isDisabled
that holds a Boolean value. You can then use the ternary operator to bind the disabled
attribute to the expression isDisabled ? true : false
. This means that if isDisabled
is true, the input field will be disabled, and if it’s false, the input field will be enabled.
How can input fields be disabled conditionally in Vue js?
In this code, the input field with the ID “confirmPassword” is conditionally disabled based on the length of the “newPassword” variable.
The v-bind:disabled directive is used to bind the “disabled” attribute of the input field to a boolean value. In this case, the boolean value is determined by the ternary operator “? : ” which checks if the length of the “newPassword” variable is equal to 0. If it is, then the input field will be disabled, otherwise it will be enabled.
To summarize, the input field with ID “confirmPassword” will be disabled if the “newPassword” variable is empty, and enabled if it contains at least one character.
Vue Js Disable input Field Conditionally Example
<div id="app">
<div class="form-group">
<label for="newPassword">Please choose a new password</label>
<input type="password" class="form-control" id="newPassword" placeholder="Password" v-model="newPassword">
</div>
<div class="form-group">
<label for="confirmPassword">Please confirm your new password</label>
<input type="password" class="form-control" id="confirmPassword" placeholder="Password"
v-model="confirmPassword" v-bind:disabled="newPassword.length === 0 ? true : false">
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
newPassword: '',
confirmPassword: ''
};
}
});
</script>
Output of Vue Js Disable input Field Conditionally
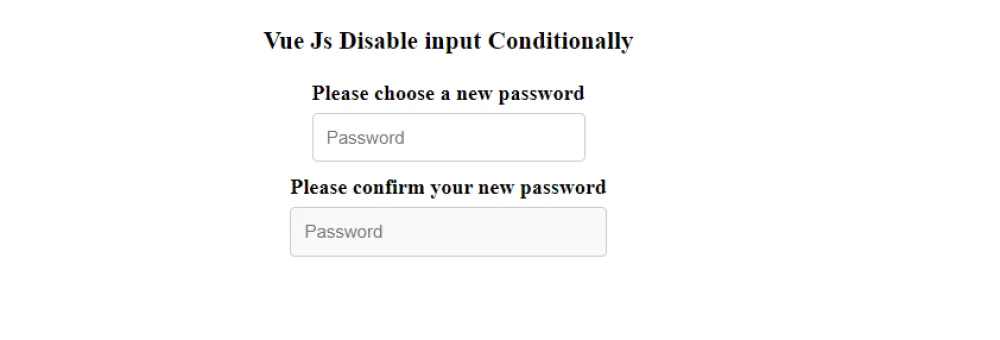