Vue Js Disable Button While Loading:In Vue.js, you can disable a button while loading by using a combination of a data property and a computed property. First, create a data property called isLoading
and set it to false
. When the button is clicked, set isLoading
to true
. Then, create a computed property called isDisabled
that returns true
if isLoading
is true
, and false
otherwise. Bind this computed property to the disabled
attribute of the button element using v-bind
. Finally, use v-on
to bind the button’s click
event to a method that sets isLoading
to true
. This will disable the button while the loading process is ongoing.
How can you Vue Js Disable Button while a loading process is taking place?
In this example, we have a button with the text “Get Data” and a loading indicator that displays when the data is being fetched. The button is disabled while the data is loading to prevent the user from clicking it multiple times and initiating multiple requests
Vue Js Disable Button While Loading Example
<div id="app">
<button :disabled="isLoading" @click="getData">Get Data</button>
<p v-if="isLoading">Loading...</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
isLoading: false,
data: null,
};
},
methods: {
async getData() {
this.isLoading = true;
setTimeout(async () => {
try {
const response = await axios.get("https://reqres.in/api/users?page=2");
this.data = response.data;
} catch (error) {
console.error(error);
} finally {
this.isLoading = false;
}
}, 5000);
},
},
});
</script>
Output of Vue Js Disable Button While Loading
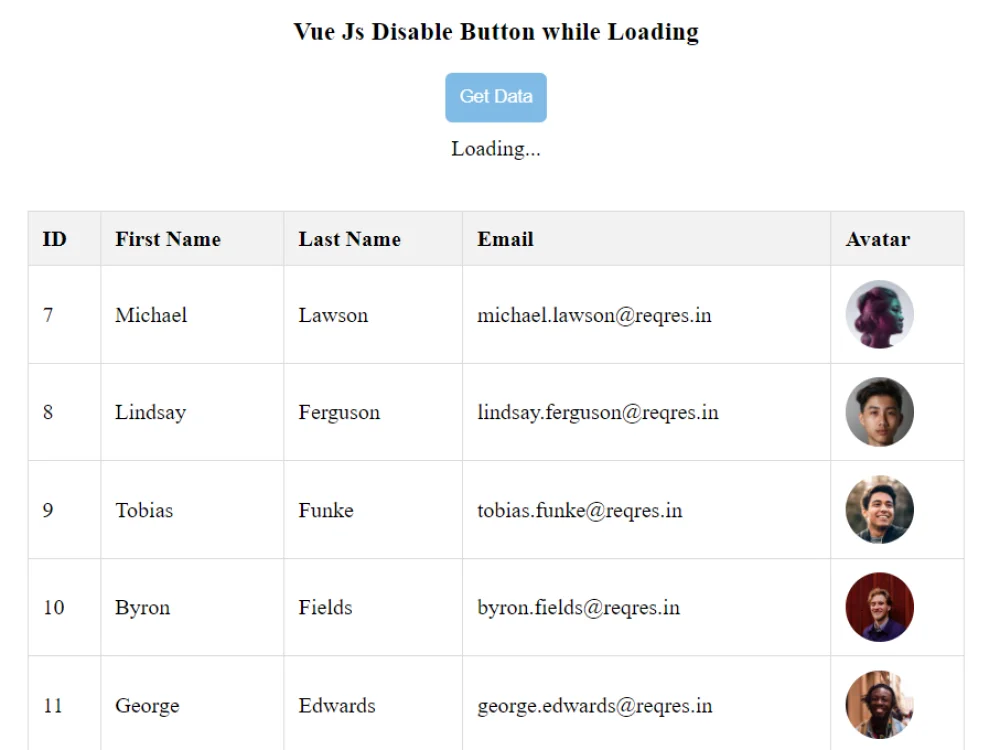