Vue Js Select Input Text On Focus:When a user focuses on a select input in Vue.js, the text inside the input is not automatically selected. However, we can use the @focus
event and a method to select the text inside the input.
To do this, we first bind the @focus
event to a method that will select the text using this.$refs.input.setSelectionRange(0, this.$refs.input.value.length)
. This method will be triggered whenever the select input is focused.
The setSelectionRange()
method takes two parameters: the start and end index of the text to be selected. In this case, we pass 0
as the start index and the length of the input value as the end index, which selects all the text inside the input.
How can you select the input text of a Vue js select input when it receives focus?
The Below code is a Vue js component that includes a label and an input element bound to a data property inputValue
using the v-model
directive.
When the input element is focused, the selectInput
method is called via the @focus
event listener. This method uses the setSelectionRange
method to select the entire contents of the input element by passing 0 as the start index and the length of the input value as the end index.
This means that when the user clicks on the input field or tabs to it, the entire content of the input field will be highlighted, making it easier for the user to start typing and replacing the existing value.
Vue Js Select Input Text On Focus Example
<div id="app">
<label for="myInput">Input:</label>
<input id="myInput" v-model="inputValue" @focus="selectInput">
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
inputValue: 'FontAwesomeicons.com'
}
},
methods: {
selectInput(event) {
event.target.setSelectionRange(0, event.target.value.length)
}
}
});
</script>
Outut of Vue Js Select Input Text on Focus:
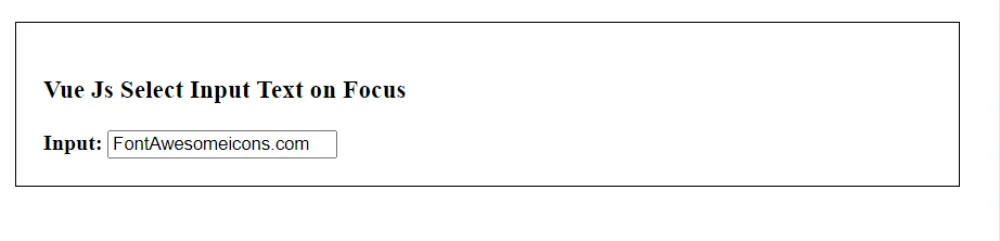