Vue Js Multiple Ternary Operator:In Vue.js, ternary operators can be used to conditionally render content in the template. A ternary operator is a shorthand way to write an if-else statement. Multiple ternary operators can be used in a nested manner to handle complex conditions. However, using too many ternary operators can make the code difficult to read and maintain.
How can I use multiple ternary operators in Vue js to conditionally render different components or elements based on multiple conditions?
This code is an example of using multiple ternary operators in Vue.js. The code creates a Vue application with two data properties: isLoggedIn
and user
.
The isLoggedIn
property is initially set to false
, and the user
property contains an object with a role
property set to 'user'
.
In the template section of the Vue app, there is a div
that displays a message depending on whether the user is logged in and their role. The message is generated using multiple nested ternary operators.
The first ternary operator checks whether isLoggedIn
is true. If it is, the second ternary operator checks whether user.role
is equal to 'admin'
. If it is, the message “Welcome, admin!” is displayed. If it isn’t, the third ternary operator checks whether user.role
is equal to 'user'
. If it is, the message “Welcome, user!” is displayed. If it isn’t, the message “Unknown user role.” is displayed.
If isLoggedIn
is false, the message “Please log in.” is displayed.
The input
element allows the user to change the user.role
property, and the button
element toggles the isLoggedIn
property between true
and false
Vue Js Multiple Ternary Operator Example
<div id="app">
<h1>Vue Js Multiple Ternary Operator Example</h1>
<div>
{{ isLoggedIn ? (user.role === 'admin' ? 'Welcome, admin!' : (user.role === 'user' ? 'Welcome, user!' : 'Unknown user role.')) : 'Please log in.' }}
</div>
<input v-model="user.role">
<button @click="isLoggedIn = !isLoggedIn">{{isLoggedIn ? 'Signout': 'LogIn'}}</button>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
isLoggedIn: false,
user: {
role: 'user'
}
}
}
})
app.mount('#app')
</script>
Output of Vue Js Multiple Ternary Operator
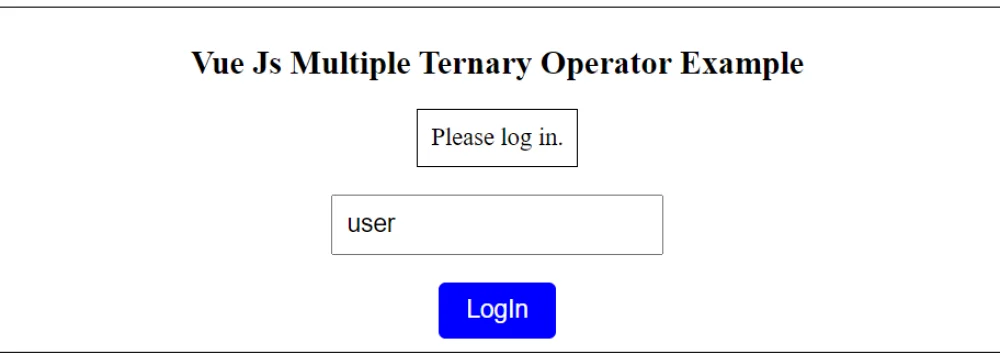