Vue Js Increase Decrease Image Size on click:Vue.js is a JavaScript framework that allows for building dynamic and reactive user interfaces. To increase or decrease the size of an image on click in Vue.js, you can define a data property to store the current size of the image, and use a method to update it when the user clicks a button. The method can then be bound to the appropriate event handler in the template. By adjusting the size value, you can apply a CSS transform to the image element and scale it up or down accordingly. This approach allows for a responsive and interactive image resizing functionality in your Vue.js application.
How can I use Vue js to increase or decrease the size of an image when a user clicks on it?
This is a Vue.js code for increasing and decreasing the image size on button click. The image is displayed using the img
tag, and the :width
binding is used to set the initial width of the image based on the imageWidth
data property.
Two buttons are provided to zoom in and zoom out the image, and their click
events are bound to the zoomIn
and zoomOut
methods respectively.
In the data
object, three properties are defined:
imageWidth
: This stores the current width of the image.maxZoomInWidth
: This defines the maximum width to which the image can be zoomed in.maxZoomOutWidth
: This defines the minimum width to which the image can be zoomed out.
The zoomIn
and zoomOut
methods are defined in the methods
object. They check if the current width of the image is within the minimum and maximum zoom limits and then increase or decrease the imageWidth
accordingly.
If the maximum zoom limit is reached when zooming in, an alert message is displayed to notify the user. Similarly, if the minimum zoom limit is reached when zooming out, an alert message is displayed to notify the user.
Vue Js Increase Decrease Image Size on click Example
<div id="app">
<img :src="'https://www.sarkarinaukriexams.com/images/post/1684081113flowers-gb1ab1f400_640.jpg'"
:width="imageWidth">
<div>
<button @click="zoomIn">Zoom In</button>
<button @click="zoomOut">Zoom Out</button>
</div>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
imageWidth: 250,
maxZoomInWidth: 500,
maxZoomOutWidth: 50,
};
},
methods: {
zoomIn() {
if (this.imageWidth >= this.maxZoomInWidth) {
alert("Highest possible level of zoom has been reached.");
} else {
this.imageWidth += 50;
}
},
zoomOut() {
if (this.imageWidth <= this.maxZoomOutWidth) {
alert("Lowest possible level of zoom has been reached.");
} else {
this.imageWidth -= 50;
}
},
},
});
app.mount('#app');
</script>
Output of Vue Js Increase Decrease Image Size on click
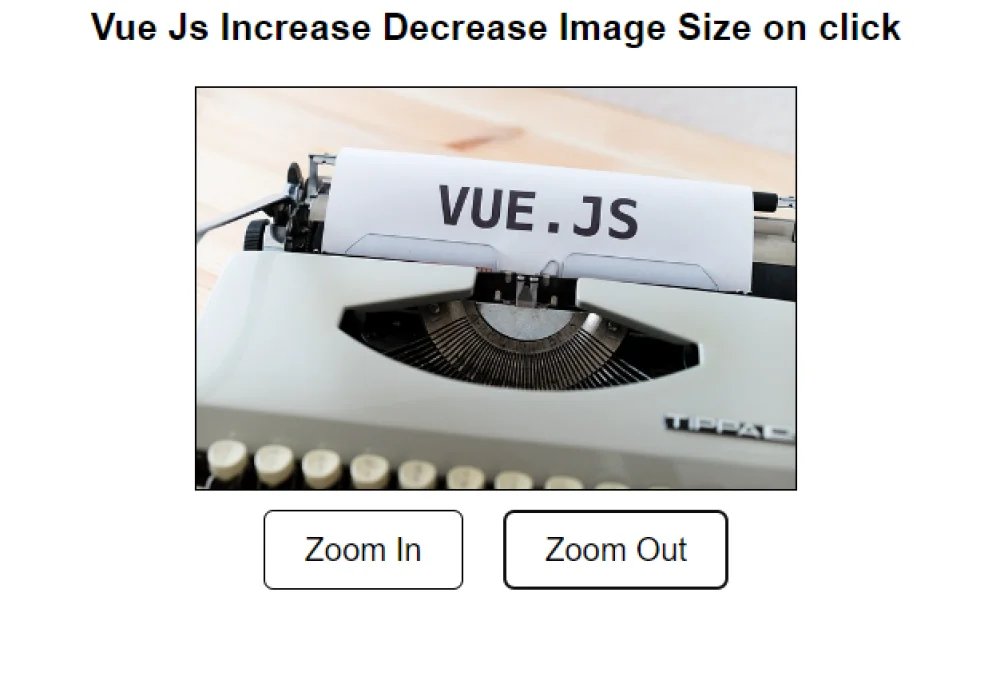