[TOC]
Vue JS Generate array of 10 random values:To generate an array of 10 random values in Vue JS using window.crypto, developers can use the built-in random number generator provided by the window.crypto object. This object provides a secure way to generate random numbers using the user’s device as a source of entropy. By calling the window.crypto.getRandomValues() method and passing in an array of 10 integers, Vue JS can generate an array of 10 random values. This can be useful for various purposes such as creating unique identifiers or generating randomized data for testing purposes
What is the code to generate an array of 10 random values using Vue JS?
This code generates an array of 10 random values in Vue JS using the window.crypto.getRandomValues()
method, which generates cryptographically secure random values. Here’s a breakdown of what the code does:
- The
data
function defines an empty array calledrandomNumbers
. - The
mounted
function is called when the component is mounted, and it calls thegenerateRandomNumbers
method to generate the random numbers. - The
generateRandomNumbers
method creates a newUint32Array
of length 10 to store the random values. - It then calls the
getRandomValues
method onwindow.crypto
to fill the array with cryptographically secure random values. - Finally, it converts the
Uint32Array
to a regular array using theArray.from()
method and assigns it to therandomNumbers
data property.
Overall, this code generates an array of 10 random values that can be accessed in the Vue template via the randomNumbers
data propert
Vue JS Generate array of 10 random values Example
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
randomNumbers: [],
};
},
mounted() {
this.generateRandomNumbers(); // Generate random numbers when the component is mounted
},
methods: {
generateRandomNumbers() {
const randomValues = new Uint32Array(10); // Generate an array of 10 random values
window.crypto.getRandomValues(randomValues); // Generate cryptographically secure random values
this.randomNumbers = Array.from(randomValues); // Convert the array to a regular array
},
},
})
</script>
Output of Vue JS Generate array of 10 random values
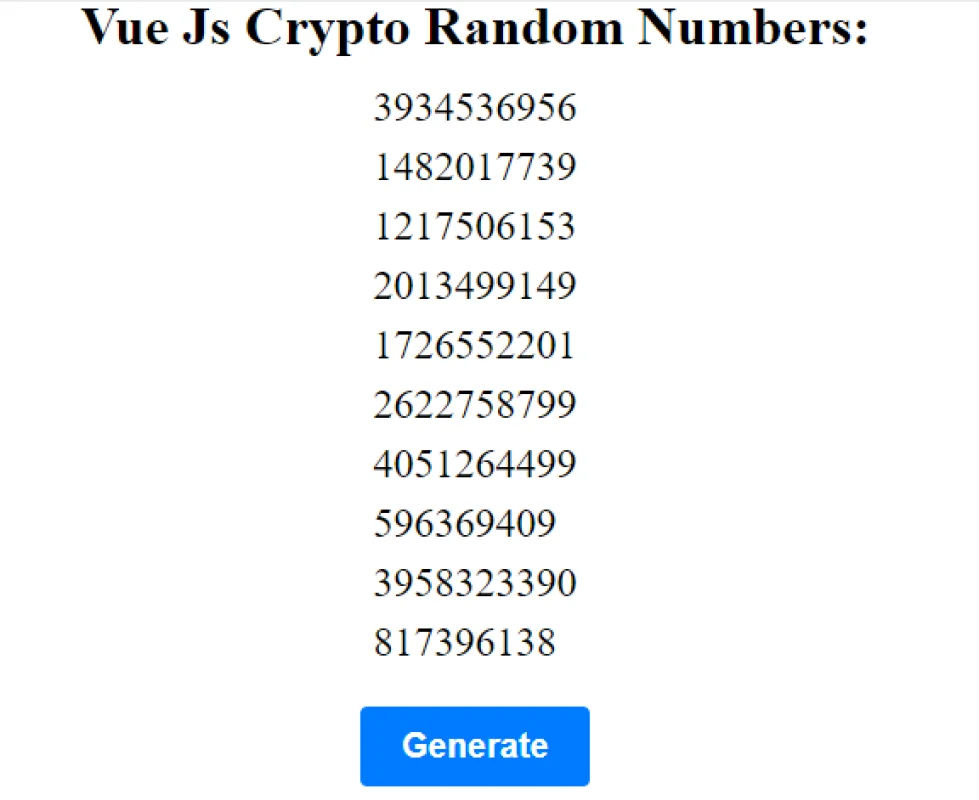