Vue Js Button :disabled multiple conditions:In Vue.js, you can disable a button based on multiple conditions using the :disabled
attribute binding. First, define the conditions as data properties in your Vue component. Then, in the button element, use the :disabled
attribute with a computed property or a method that evaluates the conditions and returns a boolean value. For example, you can use the v-bind
shorthand :
to bind the disabled
attribute to a computed property like :disabled="shouldDisableButton"
. Inside the computed property, you can check the multiple conditions and return true
or false
accordingly.
How can I Vue Js button disable based on multiple conditions?
he provided code is a Vue.js template that includes an input form for a username and password, and a submit button. The button is conditionally disabled based on multiple conditions.
The Vue instance defines the data properties username
and password
, which are bound to the input fields using v-model
. The isButtonDisabled
computed property is used to determine whether the button should be disabled.
The button is disabled if either the username or password is empty (with leading/trailing whitespace removed) or if the password length is less than 6 characters. Additional custom conditions can be added as needed.
If none of the conditions are met, the button is enabled. This allows the user to submit the form when the input requirements are satisfied.
Vue Js Button :disabled multiple conditions Example
<div id="app">
<input type="text" v-model="username" placeholder="Username">
<input type="password" v-model="password" placeholder="Password">
<button :disabled="isButtonDisabled">Submit</button>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
username: '',
password: ''
};
},
computed: {
isButtonDisabled() {
// Disable the button if username or password is empty
if (this.username.trim() === '' || this.password.trim() === '') {
return true;
}
// Disable the button if the password is less than 6 characters
if (this.password.length < 6) {
return true;
}
// Disable the button if any other custom condition is met
// For example, you can add additional validation checks here
return false; // Enable the button if none of the conditions are met
}
}
});
app.mount('#app');
</script>
Output of Vue Js Button :disabled multiple conditions
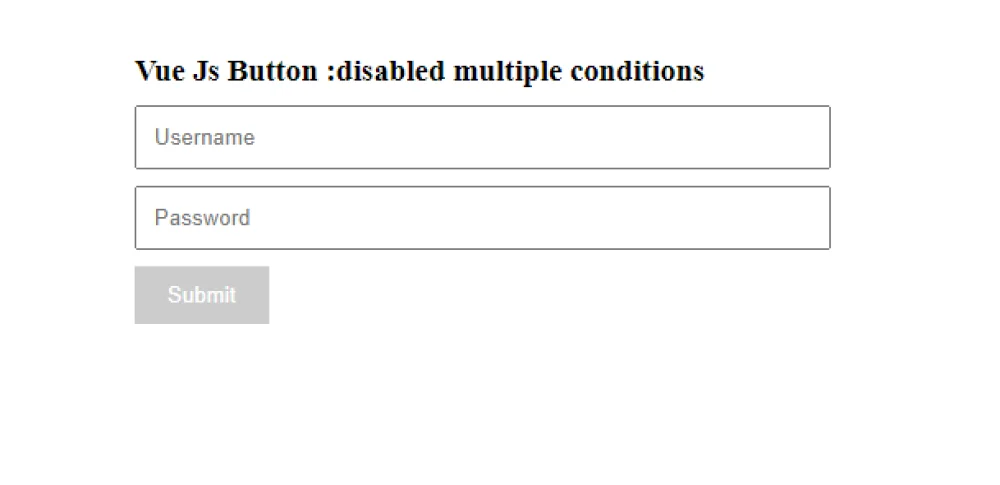