Vue Js Change Input Color Based on Value:In Vue.js, you can change the input color based on its value using conditional class binding. First, define a computed property that evaluates the value and returns a class name based on a specific condition. Then, bind this computed property to the input element’s class attribute using the :class
directive. Finally, define CSS classes for different color options in your component’s styles. By dynamically applying the appropriate class to the input element based on its value, you can change its color accordingly. This approach allows you to customize the input color based on different conditions or values, enhancing the user interface and user experience.
How can I change the color of an input field in Vue.js based on its value?
This Vue.js code sets up an input field whose background color changes based on its value. The input field is bound to the fieldValue
property in the Vue instance’s data.
The :class
and :style
bindings are used to dynamically apply CSS classes and inline styles to the input element. The fieldColorClass
computed property determines the appropriate color class based on the value of fieldValue
.
For example, if fieldValue
is between 1 and 25, the red-color
class will be applied. Similarly, different color classes are applied for different ranges. If the value doesn’t match any range, an empty string is returned as the default color class.
Vue Js Change Input Color Based on Value Example
<div id="app">
<div class="container">
<div class="field-wrapper">
<label class="field-label">Field Value</label>
<input type="text" :class="fieldColorClass" :style="{ backgroundColor: fieldColorClass }"
v-model="fieldValue" class="field-input">
</div>
<p>Value 0-25 : red color</p>
<p>Value 26-50 : Blue color</p>
<p>Value 51-75 : Yellow color</p>
<p>Value 76-100 : Green color</p>
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
fieldValue: 78 // Initial value of the field
};
},
computed: {
fieldColorClass() {
const value = this.fieldValue;
if (value >= 1 && value <= 25) {
return 'red-color';
} else if (value > 25 && value <= 50) {
return 'some-color';
} else if (value > 50 && value <= 75) {
return 'yellow-color';
} else if (value > 75 && value <= 100) {
return 'green-color';
} else {
return ''; // Default color class if value doesn't match any range
}
}
}
});
</script>
Output of Vue Js Change Input Color Based on Value
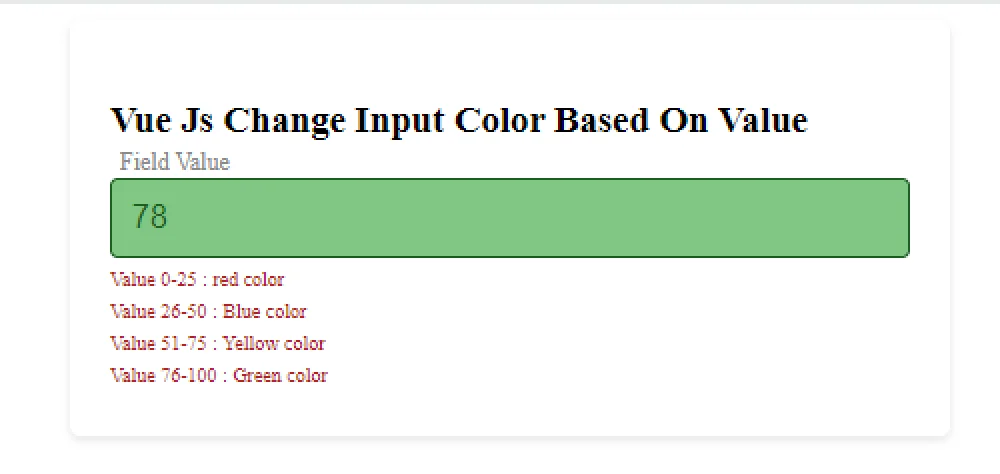