Vue Js Resize div on drag and drop:To resize a div on drag using JavaScript in Vue.js, you can utilize the built-in directives and event listeners. First, add a directive to the div element, such as v-drag
, which listens to the mousedown
event. Inside the directive, add event listeners for mousemove
and mouseup
on the document. When the mousemove
event is triggered, calculate the new width based on the mouse position and update the div’s width accordingly using reactive data. On mouseup
, remove the event listeners. This enables the div to resize dynamically as the user drags it.
How to make Vue Js Draggable Div?
This Vue.js code example demonstrates how to resize a div element by dragging it. The div element with the class “Block” contains three child elements: “Resizable1”, “Draggable”, and “Resizable2”. The width of “Resizable1” is dynamically set using the “resizable1Width” data property.
The draggable element has the “mousedown” event listener bound to the “startDrag” method, which enables dragging functionality.
When dragging occurs, the “handleDrag” method is invoked, calculating the new width of “Resizable1” based on the mouse position and the dimensions of the block. The “stopDrag” method is called when the mouse button is released, disabling dragging.
Vue Js Resize Div On Drag | Draggable Div Example
<div id="app">
<h3>Vue Js Resize div on drag and drop</h3>
<div class="Block" ref="block">
<div id="Resizable1" :style="{ width: resizable1Width + '%' }" style='background-color:#C51162;'class="ResizableBlock"></div>
<div id="Draggable" draggable="true" @mousedown="startDrag" class="Draggable"></div>
<div id="Resizable2" :style="{ width: 100 - resizable1Width + '%' }" style='background-color:#AA00FF;' class="ResizableBlock"></div>
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
draggable: false,
resizable1Width: 20,
};
},
methods: {
startDrag() {
this.draggable = true;
window.addEventListener("mousemove", this.handleDrag);
window.addEventListener("mouseup", this.stopDrag);
},
handleDrag(event) {
if (this.draggable) {
const draggableWidth = event.clientX - this.$refs.block.getBoundingClientRect().left;
const blockWidth = this.$refs.block.offsetWidth;
const newResizable1Width = (draggableWidth / blockWidth) * 100;
this.resizable1Width = newResizable1Width.toFixed(2);
}
},
stopDrag() {
this.draggable = false;
window.removeEventListener("mousemove", this.handleDrag);
window.removeEventListener("mouseup", this.stopDrag);
},
},
});
</script>
Output of Vue Js Resize Div On Drag
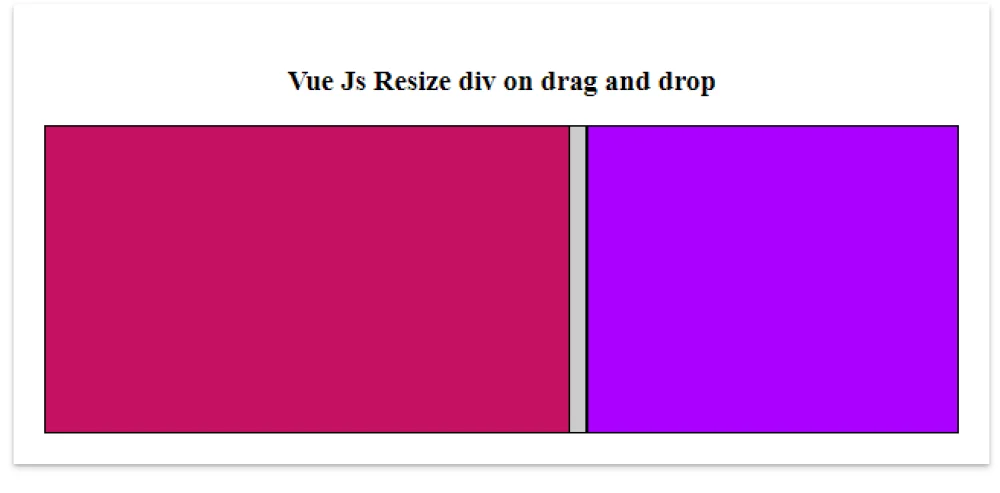