Vue Js Get Last Word of string:To get the last word of a string in Vue.js, you can use the following approach:
- Split the string into an array of words using the
split()
method, specifying the space character as the delimiter. - Retrieve the last word from the array using the
pop()
method, which removes and returns the last element. - Store the last word in a variable for further use.
How can I retrieve the last word of a string using Vue.js?
his Vue.js code creates an input field and a button. When the button is clicked, the getLastWord
method is triggered. The method splits the input string into an array of words, trims any leading or trailing whitespace, and assigns the last word to the lastWord
variable. The last word is displayed in the paragraph element using double curly braces ({{ lastWord }}
). The initial value of inputString
is “font awesome icons”.
Vue Js Get Last Word of string Example
<div id="app">
<h3>Vue Js Get Last Word of string</h3>
<input type="text" v-model="inputString" />
<button @click="getLastWord">Get Last Word</button>
<p>Last Word: {{ lastWord }}</p>
</div>
<script>
const app = new Vue({
el: "#app",
data() {
return {
inputString: 'font awesome icons',
lastWord: ''
};
},
methods: {
getLastWord() {
const words = this.inputString.trim().split(' ');
this.lastWord = words[words.length - 1];
}
}
});
</script>
Output of Vue Js Get Last Word of string
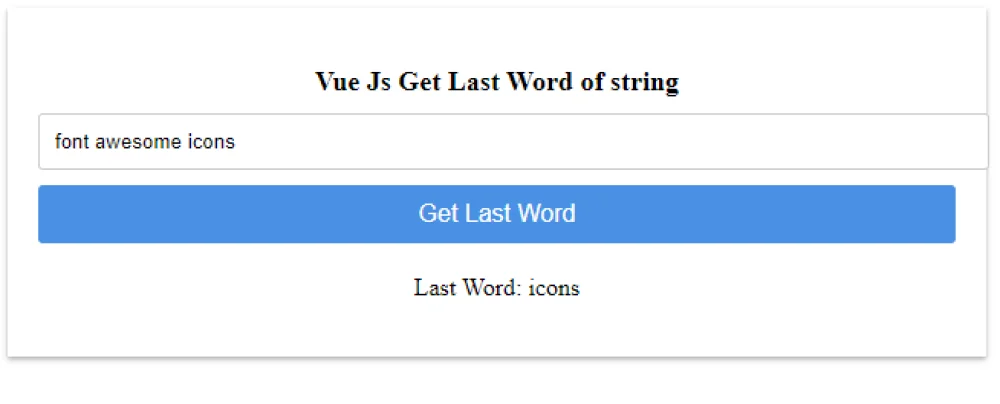