Vue Js Get First Letter of Each Word in String:In Vue.js, to get the first letter of each word in a string, you can create a computed property or a method that splits the input string into words, then maps through the words and extracts their first letters. Utilizing JavaScript’s split()
method to break the string into words and map()
to extract initial letters
How do I use Vue js to extract and display the initials (first letters) of each word in a string?
This Vue.js example demonstrates getting the first letter of each word in a string. It includes an input field to enter a string, and when text is inputted, the getFirstLetters
method splits the string into words, extracts the first letter from each word, and displays them in the firstLetters
variable. The initial text is set to “Tata Consultancy Services,” and the mounted hook ensures it’s processed on page load. The result is displayed below the input field. This code leverages Vue’s two-way data binding and event handling to achieve this functionality, making it a simple and effective way to extract and display the first letters of words in a string.
Vue Js Get First Letter of Each Word in String Example
<div id="app" class="my-app">
<h3 class="my-heading">Vue Js Get First Letter of Each Word in String Example</h3>
<input v-model="inputText" @input="getFirstLetters" placeholder="Enter a string" class="my-input" />
<p class="my-paragraph">First Letters: {{ firstLetters }}</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
inputText: "Tata Consultancy Services",
firstLetters: ""
};
},
mounted() {
this.getFirstLetters()
},
methods: {
getFirstLetters() {
const words = this.inputText.split(" ");
this.firstLetters = words.map(word => word.charAt(0)).join("");
}
}
})
</script>
Output of Vue Js Get First Letter of Each Word in String
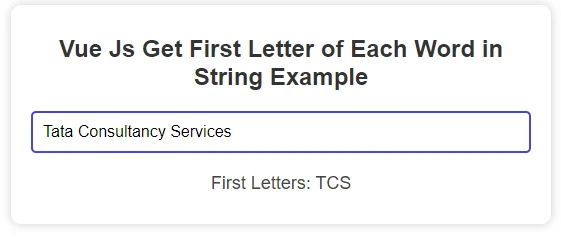