Vue Js Change Image Every 5 Seconds:Vue.js is a popular JavaScript framework that allows you to create dynamic web applications with ease. In this tutorial, we’ll walk you through a simple example of how to change an image every 5 seconds using Vue.js. We’ll provide you with the code and explain each part in deta
Vue js Change Image Every 5 Seconds: A Step-by-Step Example
In this step, we have a basic HTML structure with a placeholder for the image. We use Vue.js directives like :src
to bind the src
attribute of the image to the currentImage
property, which we’ll define in the Vue instance.
Vue Js Change Image Every 5 Seconds Example
<div id="app">
<h2>Vue Js Change Image Every 5 Seconds Example</h2>
<img :src="currentImage" alt="Image" />
</div>
In this code snippet, we first define an array of image URLs in the images
property. The currentIndex
is used to keep track of the current image being displayed, and the intervalId
is used to manage the rotation interval. We use a computed property called currentImage
to dynamically update the image source based on the currentIndex
.
The startImageRotation
method is responsible for initiating the image rotation by setting up an interval that calls the changeImage
method every 5 seconds. The stopImageRotation
method clears the interval to stop the rotation. The changeImage
method updates the currentIndex
to switch to the next image in the array.
Vue.js Image Rotation Every 5 Seconds
<script type="module">
const app = Vue.createApp({
data() {
return {
images: [
"https://www.sarkarinaukriexams.com/images/import/sne4446407201.png",
"https://www.sarkarinaukriexams.com/images/import/sne86811832.png",
"https://www.sarkarinaukriexams.com/images/import/sne10272423583.png",
"https://www.sarkarinaukriexams.com/images/import/sne1586776004.png",
"https://www.sarkarinaukriexams.com/images/import/sne20464172895.png",
// Add more image URLs as needed
],
currentIndex: 0,
intervalId: null,
};
},
computed: {
currentImage() {
return this.images[this.currentIndex];
},
},
methods: {
startImageRotation() {
this.intervalId = setInterval(this.changeImage, 5000); // 5000 milliseconds = 5 seconds
},
stopImageRotation() {
clearInterval(this.intervalId);
},
changeImage() {
this.currentIndex = (this.currentIndex + 1) % this.images.length;
},
},
created() {
this.startImageRotation(); // Start the image rotation when the component is created
},
beforeDestroy() {
this.stopImageRotation(); // Stop the interval when the component is destroyed
},
}); app.mount("#app");
</script>
Output of Vue Js Change Image Every 5 Seconds
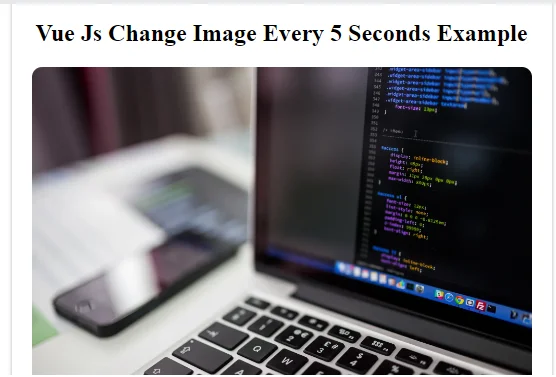
In conclusion, this Vue.js tutorial demonstrates a straightforward method to change images at 5-second intervals, adding dynamic and engaging content to web applications. By leveraging Vue.js directives and methods, developers can easily create this functionality. This example highlights the flexibility and power of Vue.js in building interactive web applications, making it a valuable tool for web development projects