Vue.js is a popular JavaScript framework for building interactive web applications. In this example, we’ll demonstrate how to perform element-wise multiplication of two arrays using Vue.js. We’ll create a simple web page that displays two input arrays and their multiplied result
Here, we have a Vue.js application that consists of three sections: “Array 1,” “Array 2,” and “Result.” You can input the values for Array 1 and Array 2, and the Result section will display the element-wise multiplication of these two arrays
Vue Js Multiple Two Array Of Element Example
<div id="app">
<h3>Vue Js Multiple Two Array of Element</h3>
<div class="custom-class">
<h2>Array 1:</h2>
{{array1}}
</div>
<div class="custom-class">
<h2>Array 2:</h2>
{{array2}}
</div>
<div class="custom-class">
<h2>Result:</h2>
{{multipliedArray}}
</div>
</div>
Implementing Element-Wise Multiplication in Vuejs
In the JavaScript part of the code, we define a Vue instance. It contains two data properties, array1
and array2
, which are initialized with sample arrays. We also have a computed property, multipliedArray
, which calculates the element-wise product of the two input arrays
Vue js Element-Wise Multiplication of Two Arrays Example
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
array1: [1, 2, 3, 4],
array2: [2, 3, 4, 5],
};
},
computed: {
multipliedArray() {
if (this.array1.length !== this.array2.length) {
console.error('Arrays must have the same length for element-wise multiplication.');
return [];
}
return this.array1.map((value, index) => value * this.array2[index]);
},
},
})
</script>
If the arrays have different lengths, the code will log an error to the console, and the result will be an empty array. However, if the lengths match, it performs the element-wise multiplication using the map
function
Output of Vue Js Multiple Two Array Of Element
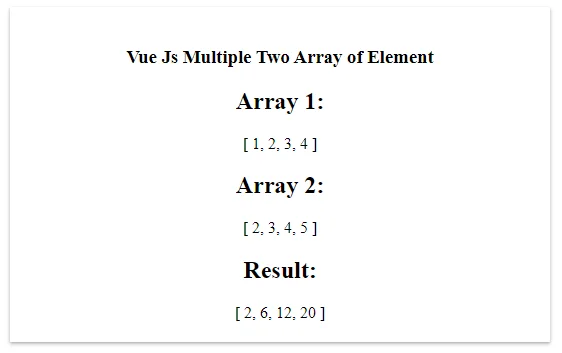
When you open this Vue.js application in a web browser, you’ll see three sections: “Array 1,” “Array 2,” and “Result.” The “Result” section will display the element-wise multiplication of the two input arrays, which, in this example, would be [2, 6, 12, 20].
In conclusion, this example demonstrates how to use Vue.js to perform element-wise multiplication of two arrays and display the result on a web page. Vue.js makes it easy to create dynamic and interactive web applications with minimal code.