Vue.js – Deselecting a Radio Button : Removing Selection from Radio Button in Vue.js” refers to the process of deselecting a previously selected radio button in a Vue.js application. In other words, it’s the action of unchecking a radio button so that no option is selected. This can be accomplished by resetting the value that the radio button is bound to in the Vue.js component’s data or by using event handling to clear the selection when a specific condition is met.
How to Uncheck a Radio Button in Vue.js?
- The root element of the application is a div with the id “app”.
- Within the root element, there is a loop (v-for directive) that iterates over the items array and creates a radio button for each item.
- The value of each radio button is bound to the current item in the loop using the “:value” directive.
- The “v-model” directive binds the selectedItem property in the data object to the radio button group, allowing the selected radio button to be dynamically updated.
- A button with the label “Uncheck” is added to the app and has a click event handler assigned to it using the “@click” directive.
- The unCheck() method, which is defined in the methods object, sets the value of selectedItem to “null” when called, effectively unchecking any previously selected radio button.
Deselecting Radio Buttons in Vue.js with a Nullifying Method
<div id="app">
<div v-for="item in items">
<input type="radio" :value="item" v-model="selectedItem">{{item}}
</div><br>
<button @click='unCheck'>Uncheck</button>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
items: ['HTML', 'CSS', 'React', 'Vue', 'Angular', 'Node', 'PHP'],
selectedItem: 'CSS',
}
},
methods: {
unCheck() {
this.selectedItem = null
}
}
});
</script>
Output of above example
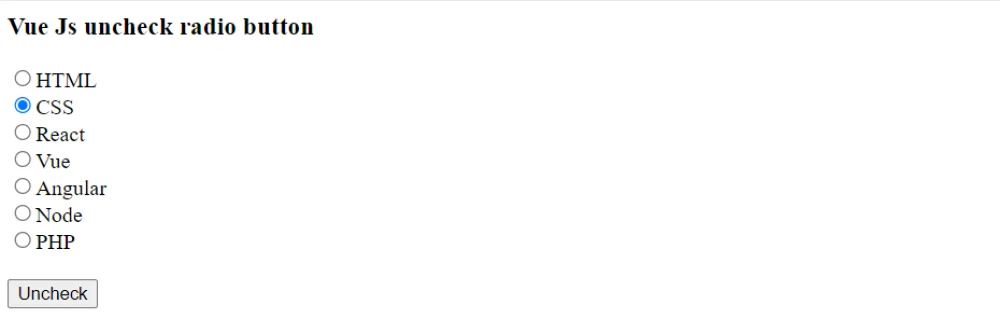