Eliminate duplicates and get unique values with the filter method and indexOf method in Vue.js: In Vue.js, one common task when working with arrays is to extract only the unique values from an array and remove any duplicates. This can be done efficiently and easily through the use of the Array.filter()
method along with the Array.indexOf()
method. This approach is great for streamlining arrays and ensuring that the data being used is accurate and up-to-date.
How to use filter method for unique value extraction in vue js
The filter method for unique value extraction in Vue.js can be used as follows:
- Define your array of elements in your Vue component’s data object.
- Create a computed property that returns the filtered array using the filter method and the indexOf method.
- In the computed property, use the filter method to loop over each element in the array.
- Within the filter method, use the indexOf method to check if the current element is the first occurrence in the array.
- If the current element is the first occurrence in the array, include it in the filtered array using the return statement.
- In your template, bind to the computed property to display the filtered array.
Array Filtering for Unique Values in Vue.js
<div id="app">
<p>Array:{{items}}</p>
<p>Get Unique Value</p>
<ul>
<li v-for="item in uniqueItems" :key="item">{{ item }}</li>
</ul>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
newItem: '',
items: ['a', 'b', 'c', 'c', 'd', 'd', 'e','f','f'],
}
},
computed: {
uniqueItems() {
return this.items.filter((item, index, self) => {
return self.indexOf(item) === index;
});
},
},
});
</script>
Output of above example
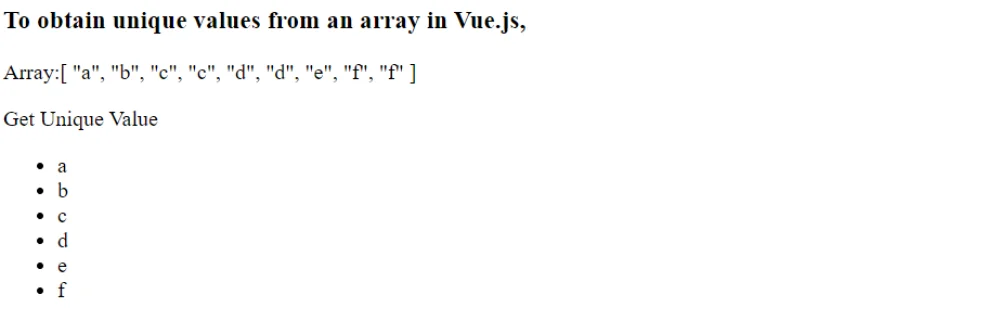