In this tutorial, we provide three examples to use Vue 3 to map an array of objects . We cover both method compositions API and options API. You can utilize these methods and try them in your project.
Example 1: Vue 3 Array Map Example
This is the first example. In this code, we use the map
function to make each element of the array uppercase. We use Vue 3 to accomplish thiis and use tryit to, edit, and customize this code according to your preferences.
Map Function in Vue Js
<script type="module">
const {createApp,ref,computed} = Vue;
createApp({
setup() {
const originalArray = ref(['Apple', 'Banana', 'Orange', 'Grapes']);
const transformedArray = computed(() => {
return originalArray.value.map(item => item.toUpperCase());
});
const transformToUppercase = () => {
originalArray.value = originalArray.value.map(item => item.toUpperCase());
};
return {
originalArray,
transformToUppercase,
};
}
}).mount("#app");
</script>
Output of Vue Js Map Array
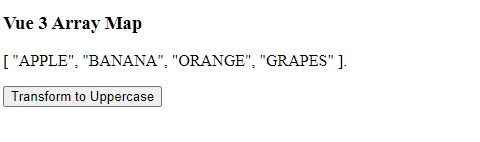
Example 2 : Vue 3 Map Array of Object Example
In this example, we use an array of objects and the map function to convert each item of the array of objects to lowercase. We can do it with Vue 3. See the code below and use it in your project
Vue Js Map Array of Object (Composition API)
<script type="module">
const {createApp,reactive} = Vue;
createApp({
setup() {
const items = reactive([
{id: 1,name: 'Andrew'},
{id: 2,name: 'Jack'},
{id: 3,name: 'Sarggy'}
]);
const modifiedItems = reactive([...items]);
const convertToUppercase = () => {
modifiedItems.forEach(item => {
item.name = item.name.toLowerCase();
});
};
return {
modifiedItems,
convertToUppercase
};
}
}).mount("#app");
</script>
Output of Vue Js Map Object
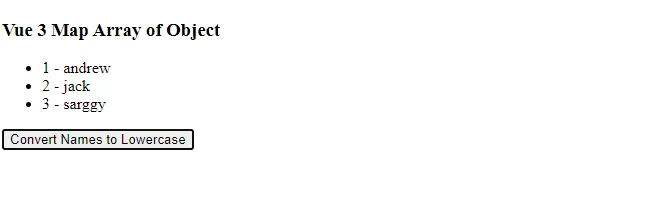
Example 3 : How to use Map function in array of Object using Vue Js (Options Api)?
In this example, we use the options API of Vue.js to map an array of objects so that you can also use this code in your project. In this code, we use Vue 2 to make every element uppercase.
Vue Js Map Array of Object (Options API)
<script type="module">
import {createApp} from 'vue'
createApp({
data() {
return {
employee: [{
'name': 'Andrew',
'email': 'andrw@gmail.com',
'role': 'Developer'
}, {
'name': 'Smith',
'email': 'smith@gmail.com',
'role': 'Marketing',
}, {
'name': 'Elon',
'email': 'elon@gmail.com',
'role': 'enterpaeneur'
}],
results: []
}
},
methods: {
myFunction() {
this.results = this.employee.map((e) => e.name.toUpperCase());
},
}
}).mount('#app')
</script>
Output of above example
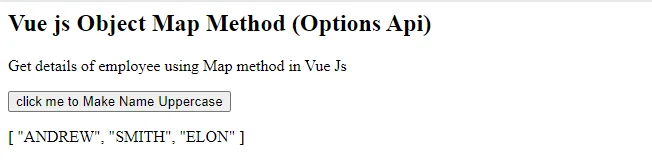