Vue Add HTML Element dynamically:In Vue, you can dynamically add HTML elements to your page by using the v-for directive and the push method. First, create an array in your Vue data that will hold the elements you want to add. Then, use the v-for directive to loop through the array and create an HTML element for each item. To add new elements dynamically, simply use the push method to add an item to the array. This will trigger a re-render of the page and display the new element(s) on the page. It’s a powerful technique for creating dynamic and reactive user interfaces in Vue.
How can you dynamically add an HTML element using Vue js?
To dynamically add an HTML element in Vue.js, use the v-for directive to loop through an array of elements and render them in the template. Then, use a method to push a new element object into the array when a button is clicked, triggering a re-render of the template.
Vue Add HTML Element dynamically Example
<div id="app">
<h3>Vue Add HTML Element dynamically</h3>
<button @click="addElement">Add Element</button>
<div v-for="(element, index) in elements" :key="index">
<h2>{{ element.title }}</h2>
<p>{{ element.description }}</p>
</div>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
elements: [
{
title: 'Element 1',
description: 'This is the first element',
}
]
}
},
methods: {
addElement() {
const newElement = {
title: 'New Element',
description: 'This is a new element',
}
this.elements.push(newElement)
},
}
})
app.mount('#app')
</script>
Output of Vue Add HTML Element dynamically
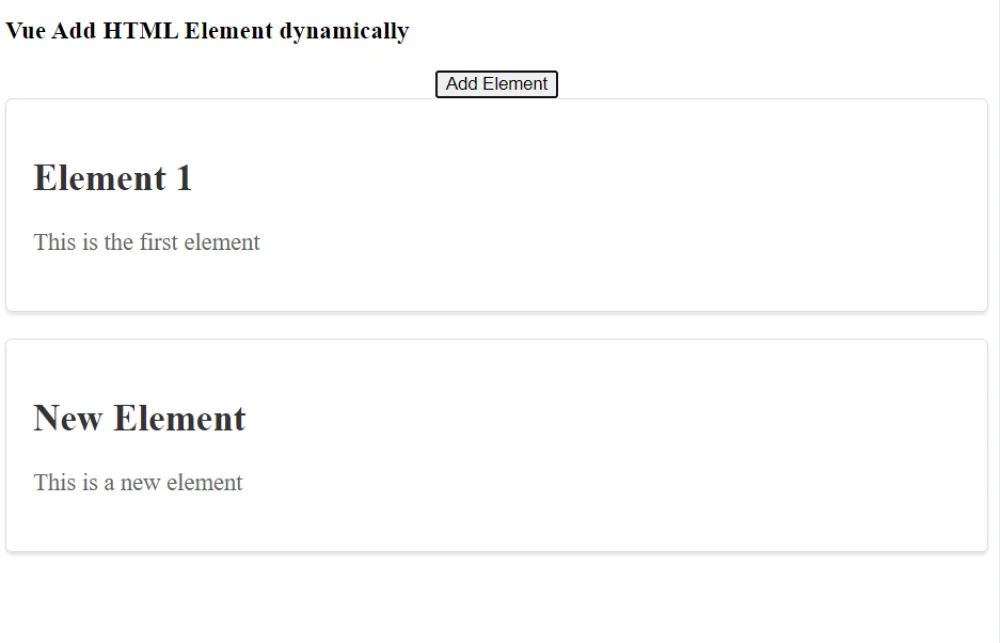