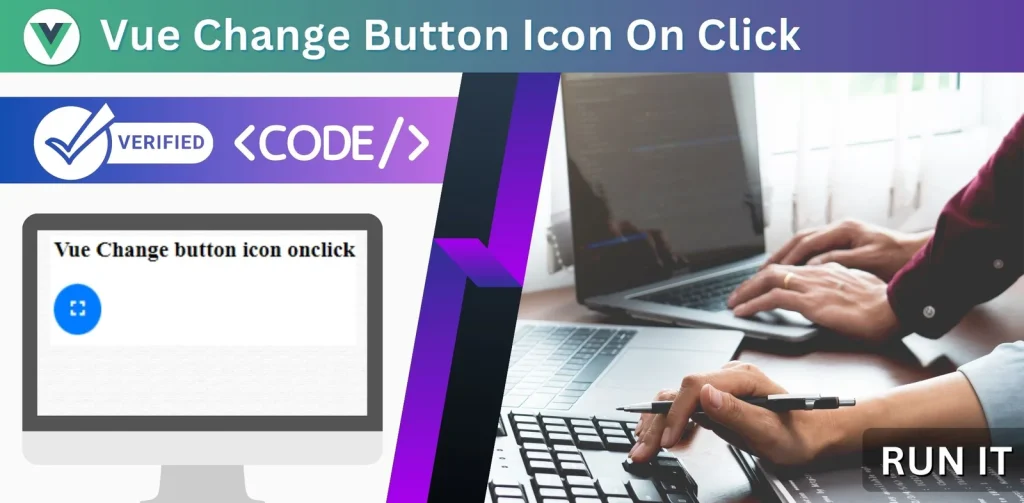
Vue Change Button Icon On Click: To change the button icon on click in Vue, you can use a data property to keep track of the button’s current state. For example, you can define a Boolean data property named isClicked that starts with a value of false.
Next, use conditional rendering to switch between the different icon states based on the value of isClicked. You can use a class binding or a v-if directive to render the appropriate icon. For instance, you can define two different classes, one for the clicked state and one for the unclicked state. Then, bind the class to the isClicked data property using a ternary operator. This way, the class will switch between the two states as the button is clicked or unclicked.
How can I change the icon of a button in Vue js when it is clicked?
The code provided demonstrates how to change the icon of a button in Vue.js when it is clicked. This is achieved by using a boolean variable “isClicked” that is toggled each time the button is clicked.
The “:class” directive is used to dynamically bind the class of the “i” tag to the “mdi-fullscreen” or “mdi-fullscreen-exit” class based on the value of “isClicked”.
Therefore, when the button is clicked, the “isClicked” variable is toggled and the class of the “i” tag changes, resulting in the icon changing from fullscreen to fullscreen-exit and vice versa.
Vue Change Button Icon On Click Example
<div id="app">
<button @click="isClicked = !isClicked">
<i :class="isClicked ? 'mdi mdi-fullscreen-exit' : 'mdi mdi-fullscreen'"></i>
</button>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
isClicked: false
}
},
});app.mount('#app');
</script>
Output of Vue Change Button Icon On Click
