Vue Class Binding Multiple Condition:Vue’s class binding feature provides a convenient way to add or remove CSS classes based on dynamic conditions in a Vue component. Using the v-bind directive, you can bind a class attribute to an expression that evaluates to a Boolean or a string of space-separated class names. This allows you to easily toggle classes based on component data or computed properties, as well as combine multiple conditions using object or array syntax. For example, you can use object syntax to conditionally apply classes based on the truthiness of a property, or array syntax to add or remove classes based on the results of multiple expressions.
How can I use Vue class binding to apply multiple conditions to a single element?
In the example, Vue class binding is used to apply multiple conditions to a single element. The v-bind:class
directive is used to dynamically apply a class to the div
element based on the values of the isActive
, isError
, and isDisabled
data properties.
Here’s an explanation of how the class binding works in this example:
- The
active
class is applied whenisActive
istrue
. - The
error
class is applied whenisError
istrue
. - The
disabled
class is applied whenisDisabled
istrue
andisError
isfalse
.
This means that the div
element can have multiple classes applied to it at the same time, depending on the values of these data properties.
To use Vue class binding to apply multiple conditions to a single element, you can use the v-bind:class
directive followed by an object containing the class names as keys and their corresponding conditions as values.
Vue Class Binding Multiple Condition Example
<div id="app">
<div class="container" v-bind:class="{
'active': isActive,
'error': isError,
'disabled': isDisabled && !isError
}">
<h3>Vue Class Binding Multiple Condition</h3>
<p>Click the buttons below to toggle the classes:</p>
<button @click="isActive = !isActive">Toggle Active</button>
<button @click="isError = !isError">Toggle Error</button>
<button @click="isDisabled = !isDisabled">Toggle Disabled</button>
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
isActive: false,
isError: false,
isDisabled: false,
};
},
});
</script>
<style>
.container {
padding: 20px;
}
.active {
background-color: lightblue;
}
.error {
color: red;
}
.disabled {
opacity: 0.5;
cursor: not-allowed;
}
</style>
Output of Vue Class Binding Multiple Condition
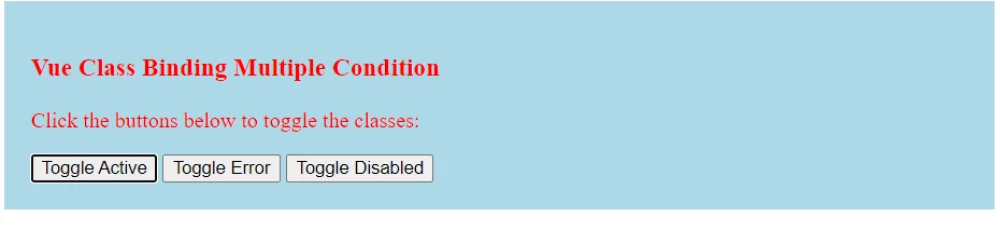