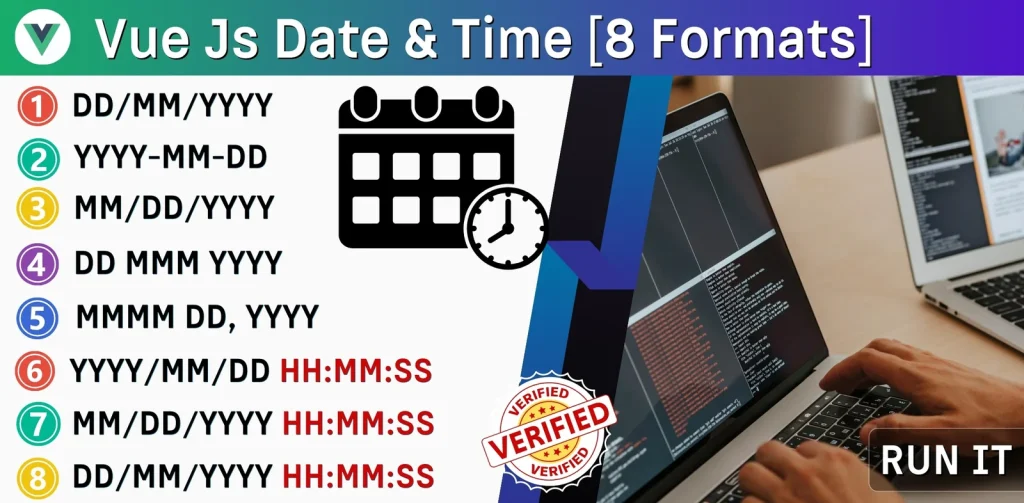
In this tutorial, we cover 8 examples of Vue.js date formatting. Throughout this guide, we’ll explore various methods to manipulate and display dates in the format you need. Starting with the toLocaleDateString
method, we’ll convert dates into the standard yyyy-mm-dd
format. As we progress, you’ll learn to format dates as YYYY-MM-DD
, followed by MM/DD/YYYY
, and then we cover more stylized dd-mmm-yyyy
. We’ll also cover how to represent dates in full month-day-year notation as MMMM DD, YYYY
, and for those needing a complete timestamp, YYYY/MM/DD HH:MM:SS
. Lastly, we’ll touch upon the dd-mm-yyyy
format.
Example 1 : tolocaledatestring format yyyy-mm-dd Vue Js
In this example, we will learn how to convert the current date format to dd/mm/yyyy using the toLocaleString method to achieve this
Vue Js Date Format dd/mm/yyyy
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
date: new Date(),
};
},
computed: {
formattedDate() {
return this.date.toLocaleString('en-GB', {
day: '2-digit',
month: '2-digit',
year: 'numeric'
});
},
},
});
</script>
Output of Vue Js Date Format DD/MM/YYYY
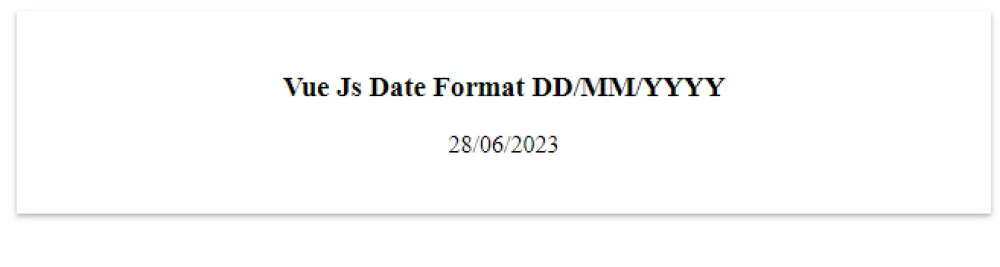
Example 2: Vue Js Date Format YYYY-MM-DD using ISOString() Method
This is the second example of this tutorial. We use the ISOString method to format the date as YYYY-MM-DD.
Vue Js Date Format YYYY-MM-DD (ISO 8601)
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
date: new Date(),
};
},
computed: {
formattedDate() {
return this.date.toISOString().split('T')[0];
},
},
});
</script>
Output of Vue Js Date Format YYYY-MM-DD
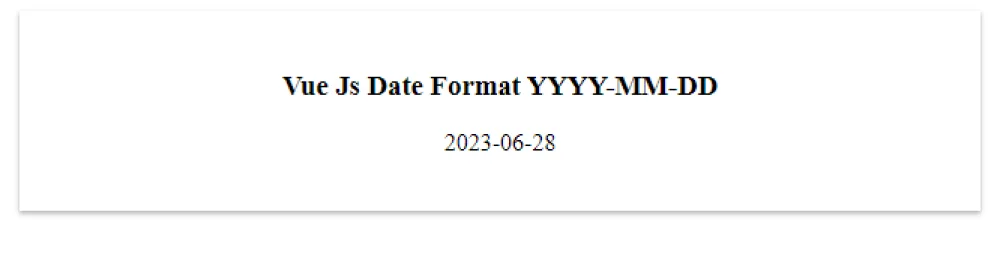
Example 3 : Date MM/DD/YYYY Format using Vue and toLocaleDateString
In this example, we demonstrate how to convert the current date into the American style format MM/DD/YYYY. We use Vue.js and a JavaScript function toLocaleDateString(‘en-us’).
Vue Js Date Format MM/DD/YYYY (American)
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
formattedDate: "",
};
},
mounted() {
const date = new Date(); // Current date
this.formattedDate = date.toLocaleDateString("en-US", {
month: "2-digit",
day: "2-digit",
year: "numeric",
});
},
});
</script>
Output of Vue Js Date Format MM/DD/YYYY
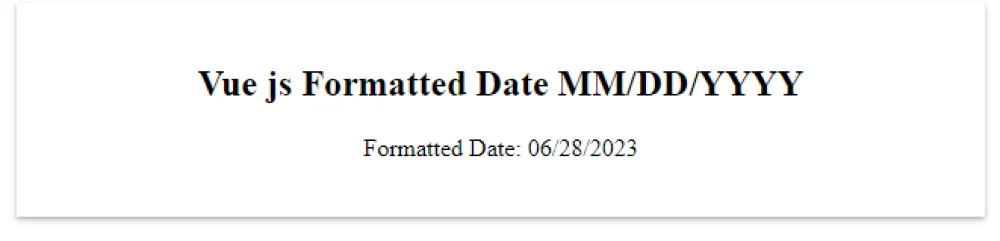
Example 4 : Vue js Convert Current Date to dd-mmm-yyyy
date format dd-mmm-yyyy
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
date: new Date(),
};
},
computed: {
formattedDate() {
const date = new Date();
return date.toLocaleDateString('en-GB', {
day: 'numeric',
month: 'short',
year: 'numeric'
}).replace(/ /g, '-');
},
},
});
</script>
Output of Vue Js Date Format DD MMM YYYY
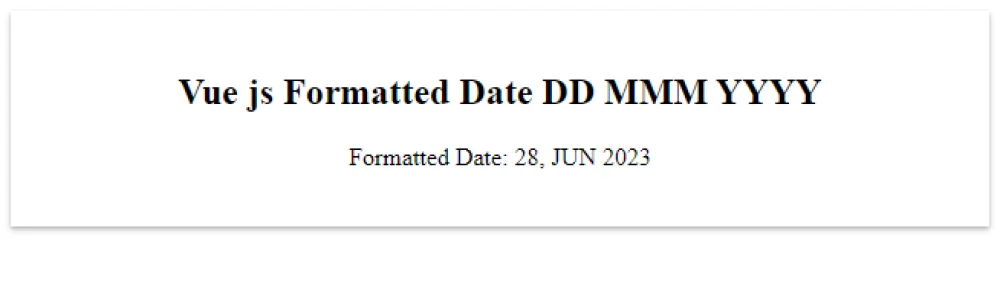
Example 5 : How can I format a date in Vuejs to display it as “MMMM DD, YYYY”?
In this example, we cover the MMMM DD, YYYY date format using the code below. For customizing and editing this code, you can use the TryIt editor.
Vue Js Date Format MMMM DD, YYYY Example
<div id="app">
<p>Formatted Date: {{ formattedDate }}</p>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
formattedDate: "",
};
},
mounted() {
this.formatDate();
},
methods: {
formatDate() {
const options = { month: "long", day: "numeric", year: "numeric" };
const date = new Date();
this.formattedDate = date.toLocaleDateString(undefined, options);
},
},
});
</script>
Output of Vue Js Date Format MMMM DD, YYYY
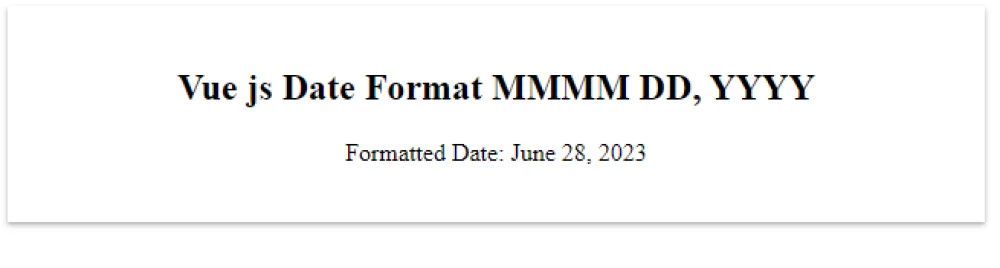
Vue Js Date Format YYYY/MM/DD HH:MM:SS (ISO 8601 with time)
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
date: new Date(),
};
},
computed: {
formattedDate() {
const d = this.date;
const dformat = [
d.getFullYear(),
d.getMonth() + 1,
d.getDate()
].join('/') + ' ' + [
d.getHours(),
d.getMinutes(),
d.getSeconds()
].join(':');
return dformat;
}
},
created() {
// Update the date every second
setInterval(() => {
this.date = new Date();
}, 1000);
}
});
</script>
Output of Vue Js Date Format YYYY/MM/DD HH:MM:SS
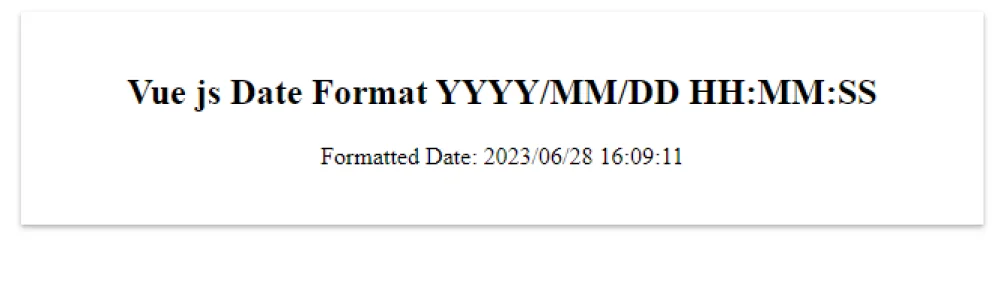
Vue Date Format dd-mm-yyyy
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
today: new Date(),
formattedToday: ''
};
},
mounted() {
this.formatDate();
},
methods: {
formatDate() {
const yyyy = this.today.getFullYear();
let mm = this.today.getMonth() + 1; // Months start at 0!
let dd = this.today.getDate();
if (dd < 10) dd = '0' + dd;
if (mm < 10) mm = '0' + mm;
this.formattedToday = dd + '-' + mm + '-' + yyyy;
}
}
});
</script>
Output of Vue Js Date format
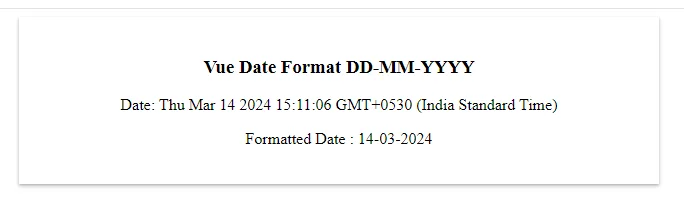
Vue Js date format MM/DD/YYYY HH:MM:SS
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
date: new Date(),
};
},
computed: {
formattedDate() {
const d = this.date;
const dformat = [
d.getMonth() + 1,
d.getDate(),
d.getFullYear(),
].join('/') + ' ' + [
d.getHours(),
d.getMinutes(),
d.getSeconds()
].join(':');
return dformat;
}
},
created() {
// Update the date every second
setInterval(() => {
this.date = new Date();
}, 1000);
}
});
</script>