Vue Get Element By Attribute:In Vue, you can use the “ref” attribute to create a reference to a specific element in your template. This reference can then be used to access the element using the “$refs” object in your component instance.
If you want to get an attribute of the element, you can use the “getAttribute()” method on the element object. This method takes the name of the attribute as its parameter and returns the value of that attribute.
How can you use Vue to get an element by its attribute?
In the mounted
hook, the myDiv
variable is assigned the reference to the div
element with the ref
attribute set to myElement
using this.$refs.myElement
. Then, the getAttribute()
method is called on the myDiv
variable with the class
attribute passed as an argument. The value of the class
attribute is then assigned to the myAttribute
data property using this.myAttribute = myDiv.getAttribute('class')
.
Therefore, the value of the myAttribute
data property will be set to the value of the class
attribute of the div
element with the ref
attribute set to myElement
.
Vue get element by attribute Example
<div id="app">
<h3>Vue Get Element by Attribute</h3>
<div ref="myElement" class="my-class" title="My Title">fontawesomeicons</div>
<pre>{{myAttribute}}</pre>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
myAttribute: ''
}
},
mounted() {
const myDiv = this.$refs.myElement;
this.myAttribute = myDiv.getAttribute('class');
}
})
app.mount('#app')
</script>
Output of Vue get element by attribute
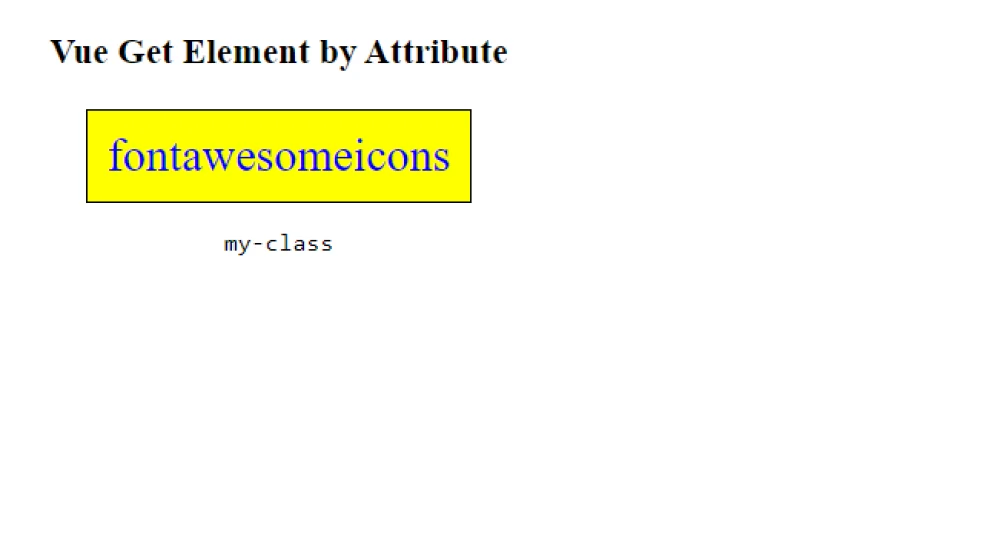