Vue Get string after specific character:In Vue, you can get the string after or before a specific character using built-in string methods such as substring
, slice
, or split
. These methods allow you to extract a portion of a string based on the position of the desired character.
To get the string after a specific character, you can use substring
or slice
to specify the starting index of the substring. Alternatively, you can use split
to split the string into an array at the specified character and then access the second element of the resulting array.
To get the string before a specific character, you can use substring
or slice
to specify the ending index of the substring. Alternatively, you can use split
to split the string into an array at the specified character and then access the first element of the resulting array.
What is the most efficient way to get the string after a specific character in Vue.js?
The Vue.js code creates an app with a data property “myString” and a method “getSubstring” that takes a string parameter and returns the substring after the “|” character. The method splits the input string by “|” and returns the second element. The app is mounted on an HTML element with the ID “app”. When the app is run, the original string is displayed along with the substring after the pipe character.
Vue Get string after specific character Example
<div id="app">
<h3>Vue Get string after specific character</h3>
<p>Original string: {{ myString }}</p>
<p>Substring after Pipline: {{ getSubstring(myString) }}</p>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
myString: 'This is question | This is Answer'
};
},
methods: {
getSubstring(str) {
return str.split('|')[1]; // Returns the second element after splitting by '|'
}
}
});app.mount("#app");
</script>
Output of Vue Get string after specific character
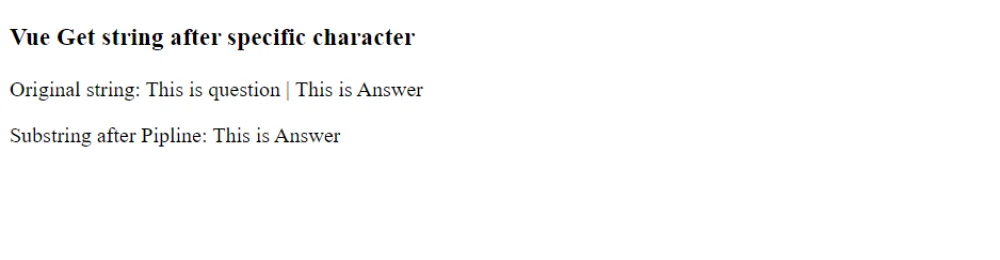
How can I get the substring before a specific character in a string using Vue.js?
This code creates a Vue.js application with a data property called “myString” set to a string. The application also has a method called “getSubstring” which takes in a string as an argument and uses the split() method to split the string by the ‘|’ character and return the first element.
Vue Get string before specific character Example
<div id="app">
<p>Original string: {{ myString }}</p>
<p>Substring Before Pipline: {{ getSubstring(myString) }}</p>
</div>
<script type="module">
const app = Vue.createApp({
data() {
return {
myString: 'Before Pipeline String | After Pipeline String'
};
},
methods: {
getSubstring(str) {
return str.split('|')[0]; // Returns the first element before splitting by '|'
}
}
});app.mount("#app");
</script>
Output of Vue Get String Before special character(|)
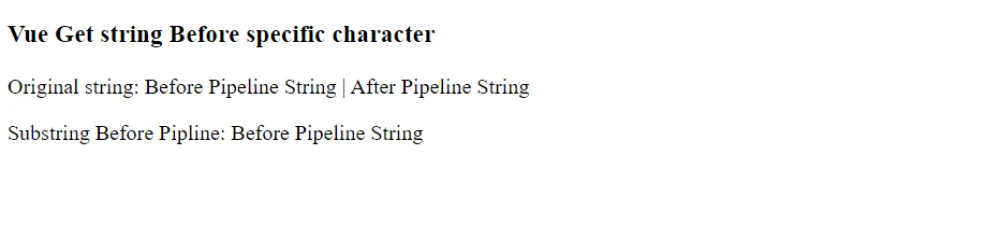