Vue Js group an array of objects by key: To group an array of objects by a key in Vue.js, you can use the reduce() method to iterate through the array and create a new object with the desired grouping. The reduce() method takes a callback function and an initial value as arguments. Within the callback function, you can use the current object’s key to check if it matches the desired grouping. If it does, you can add it to that grouping’s array. If not, you can create a new grouping with the current object and add it to the resulting object. Once all objects have been iterated through, the resulting object will contain each grouping as a property with an array of objects that match that grouping as its value.
How can you group an array of objects by a specific key in Vue.js?
The code snippet defines a method named “groupDataByCategory” which uses the reduce() method to group an array of objects by a specific key in Vue.js.
In this method, the reduce() method takes two arguments: a callback function and an initial value for the accumulator object. The callback function is executed for each object in the “data” array.
Inside the callback function, the current object’s category value is retrieved and used as a key in the accumulator object. If the key doesn’t already exist in the accumulator object, it is initialized with an empty array. The current object is then pushed into the array corresponding to its category in the accumulator object.
Finally, when all the objects have been processed, the accumulator object, which now contains the grouped data, is assigned to the “groupedData” property of the Vue instance. This allows the grouped data to be accessed in the Vue template.
Vue Group An Array Of Objects By Key Example
<div id="app">
<ul>
<li v-for="(group, category) in groupedData" :key="category">
<h3>{{ category }}</h3>
<ul>
<li v-for="item in group" :key="item.id">{{ item.name }}</li>
</ul>
</li>
</ul>
</div>
<script>
new Vue({
el: '#app',
data() {
return {
data: [
{ id: 1, name: 'John', category: 'Developer' },
{ id: 2, name: 'Jane', category: 'R & D' },
{ id: 3, name: 'Bob', category: 'Developer' },
{ id: 4, name: 'Alice', category: 'Marketing' },
{ id: 5, name: 'Mike', category: 'SEO' },
],
groupedData: {},
};
},
mounted() {
this.groupDataByCategory();
},
methods: {
groupDataByCategory() {
this.groupedData = this.data.reduce((acc, cur) => {
const category = cur.category;
if (!acc[category]) {
acc[category] = [];
}
acc[category].push(cur);
return acc;
}, {});
},
},
});
</script>
Output of Vue Js Group Array of Object by Key
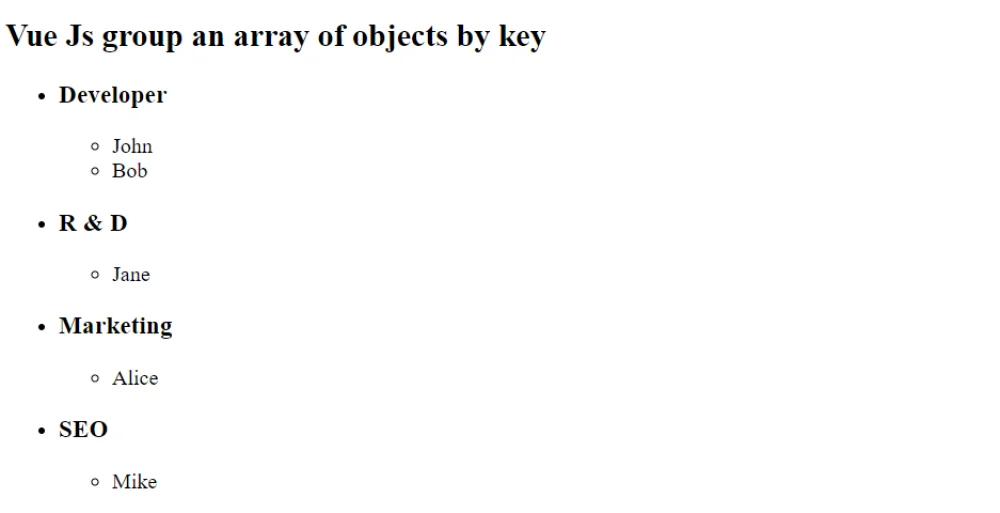