Vue Js add Item to Array if does not exist: Vue.js provides different methods to add an item to an array only if it does not already exist. Here are brief explanations of the three most common methods:
- Include method: The
includes()
method checks if an array includes a certain value. To add an item to the array if it does not exist, you can use a conditional statement withincludes()
, such asif (!myArray.includes(newValue)) { myArray.push(newValue) }
. - IndexOf method: The
indexOf()
method returns the first index at which a given element can be found in the array. To add an item to the array only if it does not exist, you can use a conditional statement withindexOf()
, such asif (myArray.indexOf(newValue) === -1) { myArray.push(newValue) }
. - Set method: The
Set
object lets you store unique values of any type. To add an item to the set only if it does not exist, you can useSet.add()
method, which only adds the value to the set if it doesn’t already exist. After that, you can convert the set back to an array, like this:myArray = [...new Set([...myArray, newValue])]
.
How can I use Vue.js to add an item to an array only if it does not already exist in the array?
This is a Vue.js script that defines a new instance of Vue with three properties: myArray, newItem, and error. myArray is an array of strings that contains three fruit names. newItem is an empty string that will be used to store a new fruit name entered by the user. error is also an empty string that will be used to display an error message if the user tries to add an item that already exists in myArray.
The script also defines a method called addItem, which is triggered when the user clicks on a button to add a new item. The method first trims the value of newItem and checks if it is not an empty string and is not already in myArray using the includes() method. If the condition is true, the new item is added to myArray using the push() method, and both newItem and error are set to an empty string. If the condition is false, an error message is assigned to error, which will be displayed on the page.
Vue Js Add Item to Array if does not exist Example
<div id="app">
<ul>
<li v-for="item in myArray" :key="item">{{ item }}</li>
</ul>
<input type="text" v-model="newItem" placeholder="Add a new item...">
<button @click="addItem">Add Item</button>
<pre v-if="error" style="color:red">{{error}}</pre>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
myArray: ['apple', 'banana', 'orange'],
newItem: '',
error: ''
};
},
methods: {
addItem() {
const item = this.newItem.trim();
if (item && !this.myArray.includes(item)) {
this.myArray.push(item);
this.newItem = '';
this.error = '';
}
else {
this.error = "Item already exist"a
}
}
}
});
</script>
Output of above example
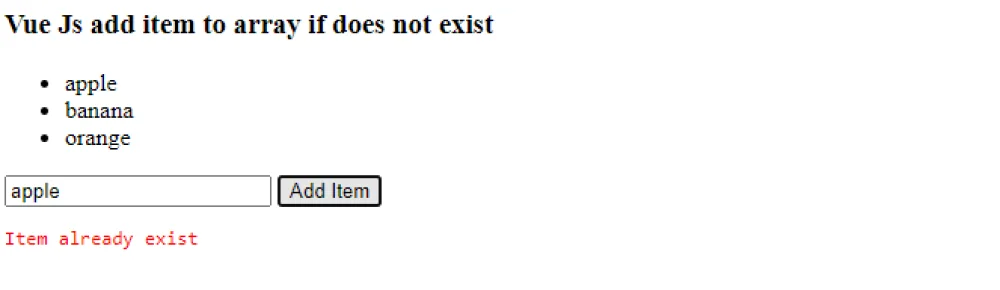
How can you use the indexOf()
method in Vue.js to add an item to an array only if it does not already exist in the array?
This code creates a Vue app that contains an array called myArray
that initially contains three strings. It also defines two other properties, newItem
and error
, which are initially empty strings.
The addItem
method is defined to add a new item to the myArray
array. First, it trims the value of newItem
and checks if it is a non-empty string that does not already exist in myArray
. If so, it adds the new item to the array, clears newItem
and sets error
to an empty string. If the new item already exists in the array or newItem
is an empty string, it sets error
to “item already exists”.
Vue Js Add Item to Array if does not exist using indexof method
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
myArray: ['Red', 'blue', 'black'],
newItem: '',
error: ''
};
},
methods: {
addItem() {
const item = this.newItem.trim();
if (item && this.myArray.indexOf(item) === -1) {
this.myArray.push(item);
this.newItem = '';
this.error = ''
}
else{
this.error = 'item alrady exist'
}
}
}
});
</script>
Vue Js Add Item to Array if does not exist using set method
<script>
export default {
data() {
return {
myArray: ['Vue', 'React', 'Angular'],
newItem: ''
}
},
methods: {
addItem() {
const item = this.newItem.trim();
if (item) {
this.myArray = [...new Set([...this.myArray, item])];
this.newItem = '';
}
}
}
}
</script>