Vue js Array every() function : every() function performs a unique operation on each element of an array. The every() method returns true if the function does so for each and every element. Every() returns false if the function returns a false value for any element. The function is not executed by the every() method for empty elements.
Vue js every() method in Array
check if all values in array are true Vue js | Example
<div id="app" class="container">
<h1>Vue js every() function</h1>
<p>When all items in an array pass a test, the every() function returns true .</p>
<p>check salary : {{salaryies}}</p>
<button class="btn btn-primary" @click="myFunction">Check Salary</button>
<p></p>
<p>{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data() {
return {
salaryies :['10000','15000','20000'],
results:''
}
},
methods:{
myFunction(){
this.results = this.salaryies.every(this.checkSalary);
},
checkSalary(salary){
return salary > 9000;
}
}
}).mount('#app')
</script>
Output of above example will look something like this –
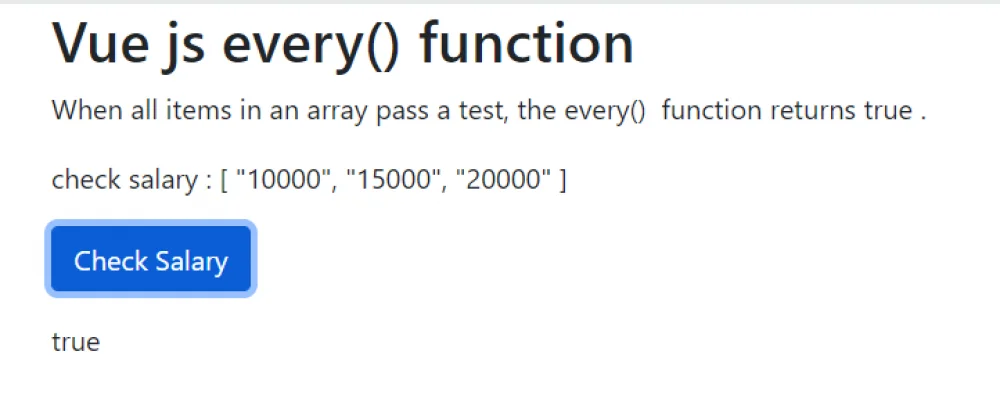
How to Determine in Vue.js Whether All Array Elements Pass a Test
check if one of values in array are false Vue js | Example
<div id="app" class="container">
<p>check result : {{marks}}</p>
<button class="btn btn-primary" @click="myFunction">Check result</button>
<p></p>
<p>{{results}}</p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data() {
return {
marks :['80','45','33'],
results:''
}
},
methods:{
myFunction(){
this.results = this.marks.every(this.checkResult);
},
checkResult(mark){
return mark > 33;
}
}
}).mount('#app')
</script>
Output of above example will look something like this –
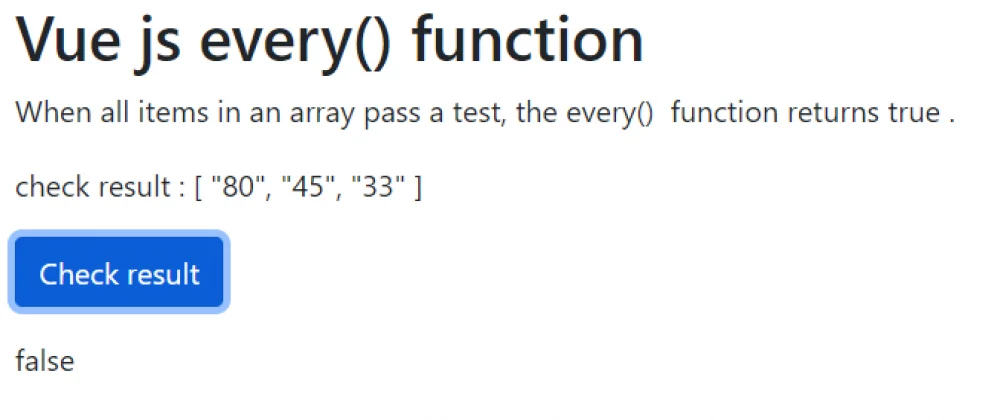