Vue js Array Filter Function : In Vue.js, the filter() method is used to filter an array based on a given function condition. The function takes an argument that represents each item in the array and returns a boolean value. If the return value is true, the item is included in the new filtered array, and if it’s false, it’s excluded. The filter() method doesn’t modify the original array but returns a new filtered array. If none of the array items meet the condition, an empty array is returned. The filter() method is useful when you need to display only specific items from an array, such as filtering out completed tasks in a to-do list or showing only certain products based on a price range.
Vue js Array Filter Even number Example
This code creates a Vue application that contains an array of numbers and a function to check if a number is even. When the myFunction
method is called, it filters the array of numbers to only include even numbers and sets the result to this filtered array. The updated result is then displayed in the HTML element with the id
of app
.
The JavaScript filter method can be used to filter the array element in Vue.js.
VueJs array filter Function Example
<div id="app" class="container">
<P>Numbers : {{numbers}}</P>
<button @click='myFunction' class='btn btn-primary'>check even number</button>
<p v-html='result'></p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
numbers : ['33','12','38','69','45','70'],
result : ''
}
},
methods:{
myFunction(){
this.result = this.numbers.filter(this.checkEvenNumber);
},
checkEvenNumber(number){
return number % 2 == 0;
}
}
}).mount('#app')
</script>
The Output of above example is given below:
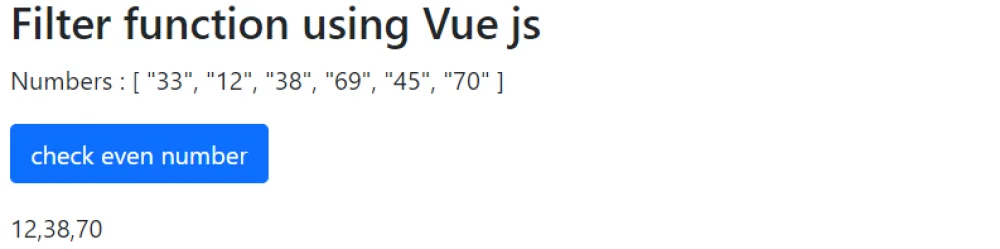
Vue js Array Filter Odd number Example
This code creates a Vue application that generates a list of numbers and filters out odd numbers from that list. The list of numbers is defined in the data
function as an array of strings. The myFunction
method is called when a button is clicked, which uses the filter()
method to remove any even numbers from the list and returns only the odd numbers.
The checkOddNumber
method is used as a callback function within the filter()
method to determine whether a given number is odd or even. If the number is odd (i.e., its remainder after division by 2 is 1), then it is returned and included in the result
variable.
VueJs array filter method Example
<div id="app" class="container">
<P>Numbers : {{numbers}}</P>
<button @click='myFunction' class='btn btn-primary'>Return odd number</button>
<p></p>
<p v-html='result'></p>
</div>
<script type="module">
import { createApp } from 'vue'
createApp({
data()
{
return{
numbers : ['32','11','37','60','41','72'],
result : ''
}
},
methods:{
myFunction(){
this.result = this.numbers.filter(this.checkOddNumber);
},
checkOddNumber(number){
return number % 2 == 1;
}
}
}).mount('#app')
</script>
The Output of above example is given below:
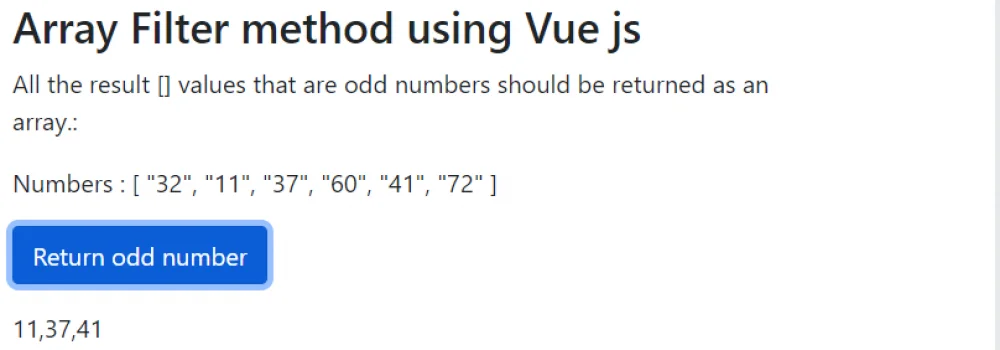