Vue.js, a progressive JavaScript framework, empowers developers to create dynamic and interactive user interfaces. In this tutorial, we’ll explore the construction of a Category-Subcategory dropdown list using Vue.js. The HTML code defines the structure, while the script section contains the Vue.js logic.
Creating a Dynamic Vue js Category Subcategory Dropdown List
The HTML structure starts with a container div with the id “app” and includes headers for clarity. Two dropdowns are defined for category and subcategory selection. The category dropdown is populated using the v-for directive, iterating over the categories array. The subcategory dropdown is initially disabled and dynamically populated based on the selected category using the v-for directive on the subcategories[selectedCategory] array.
<div id="app">
<h3>Vue js Category Subcategory Dropdown Example</h3>
<h2>Category Dropdown</h2>
<select v-model="selectedCategory" @change="updateSubcategories">
<option value="">Select Category</option>
<option v-for="category in categories" :key="category" :value="category">{{ category }}</option>
</select>
<h2>Subcategory Dropdown</h2>
<select v-model="selectedSubcategory" :disabled="!selectedCategory">
<option value="">Select Subcategory</option>
<option v-for="subcategory in subcategories[selectedCategory]" :key="subcategory" :value="subcategory">{{
subcategory
}}</option>
</select>
<div>
<h2>Selected:</h2>
<p>Category: {{ selectedCategory }}</p>
<p>Subcategory: {{ selectedSubcategory }}</p>
</div>
</div>
The selectedCategory and selectedSubcategory data properties are used to store the user’s selections. The categories array represents the available categories, and the subcategories object contains arrays of subcategories for each category.
The updateSubcategories method is defined in the Vue.js logic to reset the subcategory when the category changes, ensuring that the subcategory dropdown reflects the correct options.
<script type="module">
const app = Vue.createApp({
data() {
return {
selectedCategory: '',
selectedSubcategory: '',
categories: ['Home Decor', 'Sports Gear', 'Beauty Products', 'Toys'],
subcategories: {
'Home Decor': ['Furniture', 'Lighting', 'Wall Art', 'Candles'],
'Sports Gear': ['Running Shoes', 'Fitness Trackers', 'Sports Apparel', 'Outdoor Gear'],
'Beauty Products': ['Skincare', 'Makeup', 'Haircare', 'Fragrances'],
Toys: ['Action Figures', 'Board Games', 'Dolls', 'Educational Toys'],
},
};
},
methods: {
updateSubcategories() {
// Reset subcategory when the category changes
this.selectedSubcategory = '';
},
},
});
app.mount("#app");
</script>
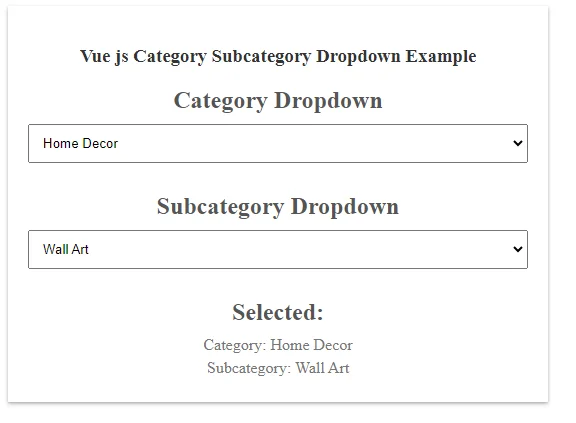
In conclusion, this Vue.js code provides a functional Category-Subcategory dropdown list with dynamic options. Users can select a category, and the corresponding subcategories are dynamically populated in the second dropdown. The selectedCategory and selectedSubcategory properties store the user’s selections, allowing for further interaction or data processing.