Vue Js Change Dynamically Background Color: To change the background color dynamically in Vue.js, you can bind a dynamic style object to the background color property of the element.
First, create a data property to hold the background color value. Then, use the v-bind:style
directive to bind a dynamic style object to the background color property of the element.
In the dynamic style object, set the background color property to the value of the data property. When the value of the data property changes, the background color of the element will also change accordingly.
How can I change the background color dynamically in Vue.js?
This code creates a simple Vue.js application that allows the user to select a color using an input field of type “color”. The selected color is stored in the Vue instance’s data property “selectedColor”.
Whenever the selected color changes, the “watch” property of the Vue instance is triggered and sets the background color of the body element to the selected color using JavaScript.
This results in a dynamic and interactive user experience where the selected color is immediately reflected in the background color of the page.
Vue Js Change Dynamically Background Color Example
<div id="app">
<div>
<p>Selected color code: {{ selectedColor }}</p>
<input type="color" v-model="selectedColor">
</div>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data: {
selectedColor: "#ffffff",
},
watch: {
selectedColor() {
document.body.style.backgroundColor = this.selectedColor;
},
},
});
</script>
Output of above example
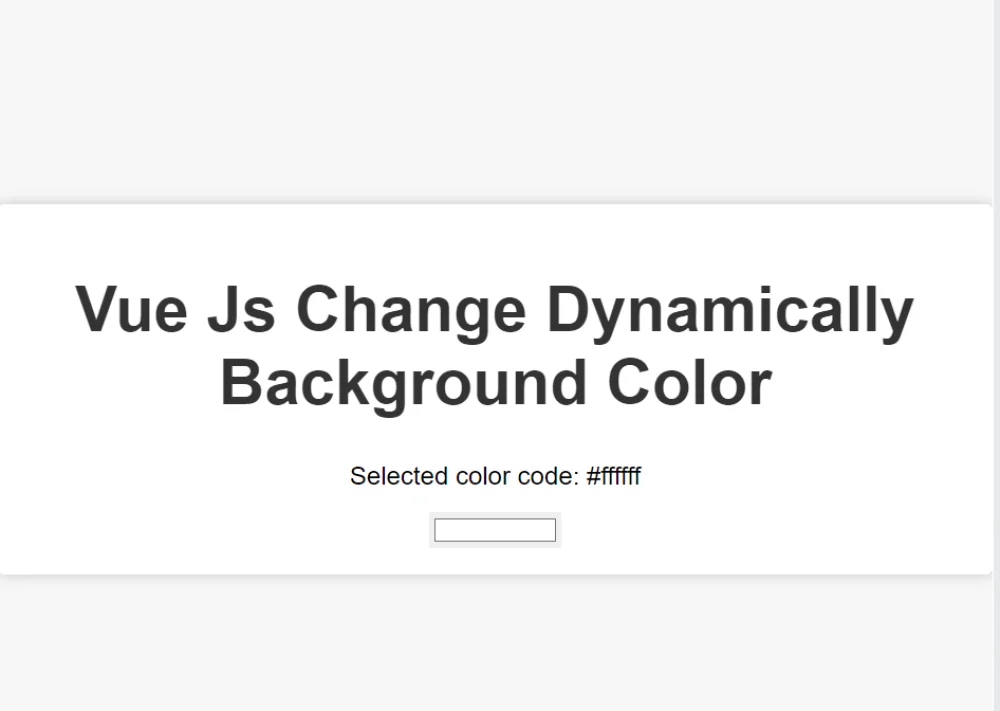